GitHub - robinchew/mvil: Android Kotlin "Virtual DOM"Android Kotlin "Virtual DOM". Contribute to robinchew/mvil development by creating an account on GitHub.
Visit Site
GitHub - robinchew/mvil: Android Kotlin "Virtual DOM"
Summary
A Android Kotlin "Virtual DOM". I wanted something like Mithril (https://mithril.js.org) and apply the Meiosis pattern (http://meiosis.js.org) for Android development.
Also inspired by Anvil (https://github.com/zserge/anvil). Hence the name.
So this abomination was born. PR welcomed.
Buzzwords:
- SPA (Single Page Application)
- Single Activity Architecture
- Functional Reactive Programming (https://github.com/paldepind/flyd)
Example
Custom Shortcuts
Where the m
, attrs
, children
comes from, it's user set.
typealias m = Virtual
fun attrs(vararg args: (View) -> Unit): ArrayList<(View) -> Unit> =
arrayListOf(*args)
fun children(vararg args: Virtual): ArrayList<Virtual> = arrayListOf(*args)
View
fun myView(activity: Context, value: MyState, actions: Actions): Virtual {
return m(::LinearLayout,
value.id,
attrs(
size(400, WRAP),
backgroundColorHex("#009999"),
elevation(1f),
margin(50),
onCrup {
println("x of ${it.x}. Y of ${it.y}")
},
onGlobalLayout {view ->
// animation.updatePositionOfView(view)
view.x = 400f
// animation.start()
}
),
children(
m(::TextView,
attrs(
size(WRAP, MATCH),
weight(1f),
layoutGravity(CENTER_VERTICAL),
padding(5),
text("${value.name} ${selectedTop} ${value.checked}"),
backgroundColorHex("#000066"),
onTouch {v, motionEvent ->
true
})),
m(::CheckBox,
attrs(
size(WRAP, WRAP),
margin(5),
layoutGravity(CENTER_VERTICAL),
focusable(false),
focusableInTouchMode(false),
clickable(false),
checked(value.checked),
onClick {
actions::toggleCheck()
}))))
}
Global State
data class MyState(val id: String, val name: String, val checked: Boolean)
Actions
Functions that changes the global state.
class Actions(val update: (f: (MyState) -> MyState) -> Unit) {
fun toggleCheck() {
update { state: MyState ->
state.copy(check=!state.checked)
}
}
}
Activity with Static View
import mvil.*
class MyActivity: Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
val renderable = RenderView(this)
renderable.sync(myView(
activity,
MyState("id123", "My Title", true),
Actions(subject::onNext)))
setContentView(renderable)
}
}
Activity with Dynamic View that updates on state change
import io.reactivex.subjects.PublishSubject
import mvil.*
class MyActivity: Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
val renderable = RenderView(this)
renderable.sync()
val subject = PublishSubject.create<(MyState) -> MyState>();
val actions = Actions(subject::onNext)))
subject.scan(
myView(activity, MyState("id123", "My Title", true), actions),
{old, new -> new(old)}
).subscribe {
renderable.sync(myView(activity, it))
}
setContentView(renderable)
}
}
Improvements
If I was better at using Kotlin features like:
- https://kotlinlang.org/api/latest/jvm/stdlib/kotlin/to.html
- https://kotlinlang.org/docs/reference/properties.html
- https://kotlinlang.org/docs/reference/type-safe-builders.html
It would have looked more like (credit to @isiahmeadows for the advice):
// EXAMPLE ONLY
// CODE BELOW DOESN'T WORK
m(::LinearLayout, id=value.id) {
size = 400 x WRAP,
backgroundColorHex = "#009999"
elevation = 1f
margin = 50
onCrup {
println("x of ${it.x}. Y of ${it.y}")
}
onGlobalLayout { view ->
value.animation.updatePositionOfView(view)
view.x = 400f
value.animation.start()
}
m(::TextView) {
attrs {
size = WRAP x MATCH
weight = 1f
layoutGravity = CENTER_VERTICAL
padding = 5
text = "${value.name} ${selectedTop} ${value.checked}"
backgroundColorHex("#000066"),
onTouch {v, motionEvent ->
val view = v.parent as LinearLayout
true
}
}
}
m(::CheckBox) {
size = WRAP x WRAP
margin = 5
layoutGravity = CENTER_VERTICAL
focusable = false
focusableInTouchMode = false
clickable = false
checked = value.checked
onClick {
toggleCheck(value.id)
}
}
}
PR welcomed.
FAQ
Q: Why don't I just use Anko?
Mvil tries to address the issue expressed in https://github.com/Kotlin/anko/issues/321.
Q: Is it fast?
A: Probably not, PR welcomed. I was more focused on laying the foundations for functional and declarative style of programming for Android.
Q: Did you try the above examples that it works?
A: Nope. It came from working code though, that was hastily rewritten.
Q: Where can I complain?
A: Find my e-mail in the commits.
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here 🔥.
- 1Kotlin | The Kotlin Blog
https://blog.jetbrains.com/kotlin/
Kotlin Archive | The JetBrains Blog
- 2Build software better, together
https://github.com/welvet/summer
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 3Build software better, together
https://github.com/themichailov/kache
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 4A Material 3 YouTube Music client for Android
https://github.com/z-huang/InnerTune
A Material 3 YouTube Music client for Android. Contribute to z-huang/InnerTune development by creating an account on GitHub.
- 5Build software better, together
https://github.com/spoptchev/scientist
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 6quasar/quasar-kotlin at master · puniverse/quasar
https://github.com/puniverse/quasar/tree/master/quasar-kotlin
Fibers, Channels and Actors for the JVM. Contribute to puniverse/quasar development by creating an account on GitHub.
- 7Kotlin Programming Language
https://kotlinlang.org/
Kotlin is a programming language that makes coding concise, cross-platform, and fun. It is Google’s preferred language for Android app development.
- 8Manga reader for Android
https://github.com/KotatsuApp/Kotatsu
Manga reader for Android. Contribute to KotatsuApp/Kotatsu development by creating an account on GitHub.
- 9A Android Application for share DeviceOwner
https://github.com/iamr0s/Dhizuku
A Android Application for share DeviceOwner. Contribute to iamr0s/Dhizuku development by creating an account on GitHub.
- 10An Illustrated Guide
https://typealias.com/start/
Are you ready to learn Kotlin programming? This book is designed to make it fun and easy to get started with Kotlin.
- 11A syntax highlighting template for the Kotlin language in LaTeX listings.
https://github.com/cansik/kotlin-latex-listing
A syntax highlighting template for the Kotlin language in LaTeX listings. - cansik/kotlin-latex-listing
- 12Simplest XML builder for Kotlin
https://github.com/qwertukg/xml-builder
Simplest XML builder for Kotlin. Contribute to qwertukg/xml-builder development by creating an account on GitHub.
- 13:sunny: Kotlin extension to pluralize and singularize strings
https://github.com/cesarferreira/kotlin-pluralizer
:sunny: Kotlin extension to pluralize and singularize strings - cesarferreira/kotlin-pluralizer
- 14Kotlin Cheat Sheet
https://speakerdeck.com/agiuliani/kotlin-cheat-sheet
Kotlin Cheat Sheet - 1 page per page version
- 15Vert.x for Kotlin
https://github.com/vert-x3/vertx-lang-kotlin
Vert.x for Kotlin. Contribute to vert-x3/vertx-lang-kotlin development by creating an account on GitHub.
- 16An intuitive Date extensions in Kotlin.
https://github.com/hotchemi/khronos
An intuitive Date extensions in Kotlin. Contribute to hotchemi/khronos development by creating an account on GitHub.
- 17:video_game: JVM game engine based on Swing/JavaFX.
https://github.com/icela/FriceEngine
:video_game: JVM game engine based on Swing/JavaFX. - icela/FriceEngine
- 18A small artefact to build json with kotlin
https://github.com/holgerbrandl/jsonbuilder
A small artefact to build json with kotlin. Contribute to holgerbrandl/jsonbuilder development by creating an account on GitHub.
- 19Kotlin Standard Functions cheat-sheet
https://medium.com/androiddevelopers/kotlin-standard-functions-cheat-sheet-27f032dd4326
A new take on a Kotlin Standard Functions Cheat-Sheet
- 20Fluent Assertion-Library for Kotlin
https://github.com/MarkusAmshove/Kluent
Fluent Assertion-Library for Kotlin. Contribute to MarkusAmshove/Kluent development by creating an account on GitHub.
- 21Mongo BSON support for kotlinx.serialization.
https://github.com/jershell/kbson
Mongo BSON support for kotlinx.serialization. Contribute to jershell/kbson development by creating an account on GitHub.
- 22A framework designed around Kotlin providing Restful HTTP Client, JDBC DSL, Loading Cache, Configurations, Validations, and more
https://github.com/sepatel/tekniq
A framework designed around Kotlin providing Restful HTTP Client, JDBC DSL, Loading Cache, Configurations, Validations, and more - sepatel/tekniq
- 23Distributed lock service | 分布式锁服务
https://github.com/Ahoo-Wang/Simba
Distributed lock service | 分布式锁服务. Contribute to Ahoo-Wang/Simba development by creating an account on GitHub.
- 24IntelliJ IDEA Community Edition & IntelliJ Platform
https://github.com/JetBrains/intellij-community
IntelliJ IDEA Community Edition & IntelliJ Platform - JetBrains/intellij-community
- 25Multiplexed, coroutine-powered reliable UDP for Kotlin using fountain codes
https://github.com/seniorjoinu/reliable-udp
Multiplexed, coroutine-powered reliable UDP for Kotlin using fountain codes - seniorjoinu/reliable-udp
- 26Firefly is an asynchronous web framework for rapid development of high-performance web application.
https://github.com/hypercube1024/firefly
Firefly is an asynchronous web framework for rapid development of high-performance web application. - hypercube1024/firefly
- 27Kotlin Multiplatform MQTT client & embeddable and standalone broker
https://github.com/davidepianca98/KMQTT
Kotlin Multiplatform MQTT client & embeddable and standalone broker - davidepianca98/KMQTT
- 28Mustache template engine in kotlin multiplatform
https://github.com/L-Briand/KTM
Mustache template engine in kotlin multiplatform. Contribute to L-Briand/KTM development by creating an account on GitHub.
- 29Simple and powerful DI for kotlin multiplatform
https://github.com/sergeshustoff/dikt
Simple and powerful DI for kotlin multiplatform. Contribute to sergeshustoff/dikt development by creating an account on GitHub.
- 30Naive one-pass recursive descent, scannerless parser framework for Kotlin
https://github.com/ParserKt/ParserKt
Naive one-pass recursive descent, scannerless parser framework for Kotlin - ParserKt/ParserKt
- 31Kotlin NBT library for kotlinx.serialization
https://github.com/BenWoodworth/knbt
Kotlin NBT library for kotlinx.serialization. Contribute to BenWoodworth/knbt development by creating an account on GitHub.
- 32Compose Multiplatform, a modern UI framework for Kotlin that makes building performant and beautiful user interfaces easy and enjoyable.
https://github.com/JetBrains/compose-multiplatform
Compose Multiplatform, a modern UI framework for Kotlin that makes building performant and beautiful user interfaces easy and enjoyable. - JetBrains/compose-multiplatform
- 33HttpMocker is a simple HTTP mocking library written in Kotlin to quickly and easily handle offline modes in your apps
https://github.com/speekha/httpmocker
HttpMocker is a simple HTTP mocking library written in Kotlin to quickly and easily handle offline modes in your apps - speekha/httpmocker
- 34A rule-based entity management library written in Kotlin
https://github.com/kciter/thing
A rule-based entity management library written in Kotlin - kciter/thing
- 35A DSL ORM library for Kotlin Multiplatform.
https://github.com/ctripcorp/SQLlin
A DSL ORM library for Kotlin Multiplatform. Contribute to ctripcorp/SQLlin development by creating an account on GitHub.
- 36YAML support for kotlinx.serialization
https://github.com/charleskorn/kaml
YAML support for kotlinx.serialization. Contribute to charleskorn/kaml development by creating an account on GitHub.
- 37Kotlin DSL http client
https://github.com/rybalkinsd/kohttp
Kotlin DSL http client. Contribute to rybalkinsd/kohttp development by creating an account on GitHub.
- 38BRVAH:Powerful and flexible RecyclerAdapter
https://github.com/CymChad/BaseRecyclerViewAdapterHelper
BRVAH:Powerful and flexible RecyclerAdapter. Contribute to CymChad/BaseRecyclerViewAdapterHelper development by creating an account on GitHub.
- 39Kwery is an SQL library for Kotlin
https://github.com/andrewoma/kwery
Kwery is an SQL library for Kotlin. Contribute to andrewoma/kwery development by creating an account on GitHub.
- 40Learn Kotlin - Best Kotlin Tutorials | Hackr.io
https://hackr.io/tutorials/learn-kotlin
Learning Kotlin? Check out these best online Kotlin courses and tutorials recommended by the programming community. Pick the tutorial as per your learning style: video tutorials or a book. Free course or paid. Tutorials for beginners or advanced learners. Check Kotlin community's reviews & comments.
- 41Kotlin for Java Developers Course
https://teamtreehouse.com/library/kotlin-for-java-developers
161-minute Android course: Kotlin was recently announced as a new official Android language! It runs on the JVM and can be used to develop Android ...
- 42Framework for quickly creating connected applications in Kotlin with minimal effort
https://github.com/ktorio/ktor
Framework for quickly creating connected applications in Kotlin with minimal effort - ktorio/ktor
- 43Kotlin Web Framework for the JVM
https://github.com/TinyMission/kara
Kotlin Web Framework for the JVM. Contribute to TinyMission/kara development by creating an account on GitHub.
- 44A minimalist web framework for building REST APIs in Kotlin/Java.
https://github.com/SeunAdelekan/Kanary
A minimalist web framework for building REST APIs in Kotlin/Java. - SeunAdelekan/Kanary
- 45sponge is a website crawler and links downloader command-line tool
https://github.com/spypunk/sponge
sponge is a website crawler and links downloader command-line tool - spypunk/sponge
- 46Read-only mirror of Grayjay repo for issue tracking
https://github.com/futo-org/grayjay-android
Read-only mirror of Grayjay repo for issue tracking - futo-org/grayjay-android
- 47Doodle | Doodle
https://nacular.github.io/doodle/
A pure Kotlin, UI framework for the Web and Desktop
- 48A Kotlin multiplatform library for arbitrary precision arithmetics
https://github.com/ionspin/kotlin-multiplatform-bignum
A Kotlin multiplatform library for arbitrary precision arithmetics - ionspin/kotlin-multiplatform-bignum
- 49Kotlin Discussions
https://discuss.kotlinlang.org/
Kotlin is a concise multiplatform language developed by JetBrains.
- 50http4k - a functional Kotlin HTTP toolkit
https://www.http4k.org
The Functional toolkit for Kotlin HTTP applications
- 51Stove: The easiest way of writing e2e tests for your JVM back-end API with Kotlin
https://github.com/Trendyol/stove
Stove: The easiest way of writing e2e tests for your JVM back-end API with Kotlin - Trendyol/stove
- 52Kotlin Notebook - IntelliJ IDEs Plugin | Marketplace
https://plugins.jetbrains.com/plugin/16340-kotlin-notebook
Kotlin notebooks are interactive worksheets with rich outputs that allow you to explore and experiment with your Kotlin code without additional environment setup. The...
- 53LinkedIn Login, Sign in | LinkedIn
https://www.linkedin.com/groups/7417237/profile
Login to LinkedIn to keep in touch with people you know, share ideas, and build your career.
- 54An Android application for streaming music from YouTube Music.
https://github.com/vfsfitvnm/ViMusic
An Android application for streaming music from YouTube Music. - vfsfitvnm/ViMusic
- 55Kotlin for Android Developers
https://leanpub.com/kotlin-for-android-developers
Learn how Kotlin can make your life easier by creating a complete Android App from the ground up, step by step, while learning the language basics.
- 56拷贝漫画的第三方APP,优化阅读/下载体验
https://github.com/fumiama/copymanga
拷贝漫画的第三方APP,优化阅读/下载体验. Contribute to fumiama/copymanga development by creating an account on GitHub.
- 57Adds themed icons to Lawnchair.
https://github.com/LawnchairLauncher/lawnicons
Adds themed icons to Lawnchair. Contribute to LawnchairLauncher/lawnicons development by creating an account on GitHub.
- 58Kotlin DSL for HTML
https://github.com/Kotlin/kotlinx.html
Kotlin DSL for HTML. Contribute to Kotlin/kotlinx.html development by creating an account on GitHub.
- 59自动跳过APP开屏广告
https://github.com/GuoXiCheng/SKIP
自动跳过APP开屏广告. Contribute to GuoXiCheng/SKIP development by creating an account on GitHub.
- 60A library that tests if the implementation of a REST-API meets its specification.
https://github.com/codecentric/hikaku
A library that tests if the implementation of a REST-API meets its specification. - codecentric/hikaku
- 61Kotlin Coroutines
https://kt.academy/book/coroutines
A practical book dedicated to Kotlin coroutines, explaining built-in support, kotlinx.coroutines library and the best practices for real-life projects.
- 62A Kotlin compiler plugin that removes the `copy` method of data classes.
https://github.com/AhmedMourad0/no-copy
A Kotlin compiler plugin that removes the `copy` method of data classes. - AhmedMourad0/no-copy
- 63Hands-On-Design-Patterns-with-Kotlin, published by Packt
https://github.com/AlexeySoshin/Hands-on-Design-Patterns-with-Kotlin
Hands-On-Design-Patterns-with-Kotlin, published by Packt - AlexeySoshin/Hands-on-Design-Patterns-with-Kotlin
- 64Pure Kotlin GraphQL implementation
https://github.com/aPureBase/KGraphQL
Pure Kotlin GraphQL implementation. Contribute to aPureBase/KGraphQL development by creating an account on GitHub.
- 65A curated list of companies using Kotlin
https://github.com/vinigmoraes/awesome-kotlin-jobs
A curated list of companies using Kotlin. Contribute to vinigmoraes/awesome-kotlin-jobs development by creating an account on GitHub.
- 66Small library to parse and work with recipes from Chefkoch.de
https://github.com/iotacb/ChefkochAPI
Small library to parse and work with recipes from Chefkoch.de - iotacb/ChefkochAPI
- 67An acceptance test library for Kotlin
https://github.com/dmcg/konsent
An acceptance test library for Kotlin. Contribute to dmcg/konsent development by creating an account on GitHub.
- 68gRPC Kotlin Coroutines, Protobuf DSL, Scripting for Protoc
https://github.com/marcoferrer/kroto-plus
gRPC Kotlin Coroutines, Protobuf DSL, Scripting for Protoc - marcoferrer/kroto-plus
- 69Modern gRPC back-end development framework base on JVM.
https://github.com/ButterCam/sisyphus
Modern gRPC back-end development framework base on JVM. - ButterCam/sisyphus
- 70Alibaba Java Coding Guidelines pmd implements and IDE plugin
https://github.com/alibaba/p3c
Alibaba Java Coding Guidelines pmd implements and IDE plugin - alibaba/p3c
- 71Kotlin DSL for Selenium. Provide a possibility to write tests in Kotlin type-safe builders style
https://github.com/qwertukg/SeleniumBuilder
Kotlin DSL for Selenium. Provide a possibility to write tests in Kotlin type-safe builders style - qwertukg/SeleniumBuilder
- 72AnkiDroid: Anki flashcards on Android. Your secret trick to achieve superhuman information retention.
https://github.com/ankidroid/Anki-Android
AnkiDroid: Anki flashcards on Android. Your secret trick to achieve superhuman information retention. - ankidroid/Anki-Android
- 73Painless Mobile UI Automation
https://github.com/mobile-dev-inc/maestro
Painless Mobile UI Automation. Contribute to mobile-dev-inc/maestro development by creating an account on GitHub.
- 74Bitwarden mobile app for Android.
https://github.com/bitwarden/android
Bitwarden mobile app for Android. Contribute to bitwarden/android development by creating an account on GitHub.
- 75REST framework written in pure Kotlin
https://github.com/gimlet2/kottpd
REST framework written in pure Kotlin. Contribute to gimlet2/kottpd development by creating an account on GitHub.
- 76Kotlin - freeCodeCamp.org
https://www.freecodecamp.org/news/tag/kotlin/
Browse thousands of programming tutorials written by experts. Learn Web Development, Data Science, DevOps, Security, and get developer career advice.
- 77DNS over HTTPS / DNS over Tor / DNSCrypt client, WireGuard proxifier, firewall, and connection tracker for Android.
https://github.com/celzero/rethink-app
DNS over HTTPS / DNS over Tor / DNSCrypt client, WireGuard proxifier, firewall, and connection tracker for Android. - celzero/rethink-app
- 78A Hanime1.me Application for Android.
https://github.com/YenalyLiew/Han1meViewer
A Hanime1.me Application for Android. Contribute to YenalyLiew/Han1meViewer development by creating an account on GitHub.
- 79Introduction to Kotlin Programming
https://shop.oreilly.com/product/0636920052982.do
Kotlin 1.0 was released in February 2016, and since that time it’s been embraced by developers around the world, especially those within the Android community. This course provides an easy … - Selection from Introduction to Kotlin Programming [Video]
- 80Advanced Kotlin Programming
https://shop.oreilly.com/product/0636920052999.do
Designed for developers who already have a basic understanding of Kotlin, this video course examines some of the additional major features that make the language highly extensible and unique compared … - Selection from Advanced Kotlin Programming [Video]
- 81Kotlin port of RandomGen
https://github.com/EranBoudjnah/RandomGenKt
Kotlin port of RandomGen. Contribute to EranBoudjnah/RandomGenKt development by creating an account on GitHub.
- 82A Kotlin framework for building web applications in Javascript.
https://github.com/jean79/yested
A Kotlin framework for building web applications in Javascript. - jean79/yested
- 83🚀 The Rapid and Delightful Kotlin Web Framework. Easy, elegant, and productive!
https://github.com/alpas/alpas
🚀 The Rapid and Delightful Kotlin Web Framework. Easy, elegant, and productive! - alpas/alpas
- 84Head First Kotlin
https://www.oreilly.com/library/view/head-first-kotlin/9781491996683/
What will you learn from this book? Head First Kotlin is a complete introduction to coding in Kotlin. This hands-on book helps you learn the Kotlin language with a unique … - Selection from Head First Kotlin [Book]
- 85Balin is an automation library for Kotlin. It's basically a Selenium-WebDriver wrapper inspired by Geb.
https://github.com/EPadronU/balin
Balin is an automation library for Kotlin. It's basically a Selenium-WebDriver wrapper inspired by Geb. - EPadronU/balin
- 86Kotlin DSL for Junit5
https://github.com/neworld/kupiter
Kotlin DSL for Junit5. Contribute to neworld/kupiter development by creating an account on GitHub.
- 87🧩 Patches for ReVanced
https://github.com/ReVanced/revanced-patches
🧩 Patches for ReVanced. Contribute to ReVanced/revanced-patches development by creating an account on GitHub.
- 88Kotlin multiplatform / multi-format serialization
https://github.com/Kotlin/kotlinx.serialization
Kotlin multiplatform / multi-format serialization - GitHub - Kotlin/kotlinx.serialization: Kotlin multiplatform / multi-format serialization
- 89IdeaVim – A Vim engine for JetBrains IDEs
https://github.com/JetBrains/ideavim
IdeaVim – A Vim engine for JetBrains IDEs. Contribute to JetBrains/ideavim development by creating an account on GitHub.
- 90:iphone: Home Assistant Companion for Android
https://github.com/home-assistant/android
:iphone: Home Assistant Companion for Android. Contribute to home-assistant/android development by creating an account on GitHub.
- 91Html templating library for kotlin
https://github.com/phenax/h
Html templating library for kotlin. Contribute to phenax/h development by creating an account on GitHub.
- 92Functional Programming in Kotlin
https://www.manning.com/books/functional-programming-in-kotlin
Master techniques and concepts of functional programming to deliver safer, simpler, and more effective Kotlin code.</b> In Functional Programming in Kotlin</i> you will learn: Functional programming techniques for real-world applications</li> Write combinator libraries</li> Common structures and idioms in functional design</li> Simplicity and modularity (and fewer bugs!)</li> </ul> Functional Programming in Kotlin</i> is a reworked version of the bestselling Functional Programming in Scala</i>, with all code samples, instructions, and exercises translated into the powerful Kotlin language. In this authoritative guide, you’ll take on the challenge of learning functional programming from first principles. Complex concepts are demonstrated through exercises that you’ll love to test yourself against. You’ll start writing Kotlin code that’s easier to read, easier to reuse, better for concurrency, and less prone to bugs and errors.
- 93A nice parser combinator library for Kotlin
https://github.com/h0tk3y/better-parse
A nice parser combinator library for Kotlin. Contribute to h0tk3y/better-parse development by creating an account on GitHub.
- 94Lightweight JavaFX Framework for Kotlin
https://github.com/edvin/tornadofx
Lightweight JavaFX Framework for Kotlin. Contribute to edvin/tornadofx development by creating an account on GitHub.
- 95Android application to analyze WiFi signals.
https://github.com/VREMSoftwareDevelopment/WiFiAnalyzer
Android application to analyze WiFi signals. Contribute to VREMSoftwareDevelopment/WiFiAnalyzer development by creating an account on GitHub.
- 96A Spark DSL in idiomatic kotlin // dependency: com.sparkjava:spark-kotlin:1.0.0-alpha
https://github.com/perwendel/spark-kotlin
A Spark DSL in idiomatic kotlin // dependency: com.sparkjava:spark-kotlin:1.0.0-alpha - perwendel/spark-kotlin
- 97Spring support libraries for Kotlin
https://github.com/MarioAriasC/KotlinPrimavera
Spring support libraries for Kotlin. Contribute to MarioAriasC/KotlinPrimavera development by creating an account on GitHub.
- 98A shadowsocks client for Android
https://github.com/shadowsocks/shadowsocks-android
A shadowsocks client for Android. Contribute to shadowsocks/shadowsocks-android development by creating an account on GitHub.
- 99Mathematical expression engine written in Kotlin, running on JVM.
https://github.com/vittee/kformula
Mathematical expression engine written in Kotlin, running on JVM. - GitHub - vittee/kformula: Mathematical expression engine written in Kotlin, running on JVM.
- 100Test the shape of your functions!
https://github.com/krzema12/vis-assert
Test the shape of your functions! Contribute to krzema12/vis-assert development by creating an account on GitHub.
- 101Thunderbird for Android – Open Source Email App for Android (fka K-9 Mail)
https://github.com/thunderbird/thunderbird-android
Thunderbird for Android – Open Source Email App for Android (fka K-9 Mail) - thunderbird/thunderbird-android
- 102A library to generate HTML in Kotlin.
https://github.com/celtric/kotlin-html
A library to generate HTML in Kotlin. Contribute to celtric/kotlin-html development by creating an account on GitHub.
- 103A web crawling framework written in Kotlin
https://github.com/brianmadden/krawler
A web crawling framework written in Kotlin. Contribute to brianmadden/krawler development by creating an account on GitHub.
- 104Kotlin in Action, Second Edition
https://www.manning.com/books/kotlin-in-action-second-edition
Expert guidance and amazing examples from Kotlin core developers! It’s everything you need to get up and running fast.</b> Kotlin in Action, Second Edition</i> takes you from language basics to building production-quality applications that take advantage of Kotlin’s unique features. Discover how the language handles everything from statements and functions to classes and types, and the unique features that make Kotlin programming so seamless. In Kotlin in Action, Second Edition</i> you will learn: Kotlin statements and functions, and classes and types</li> Functional programming on the JVM</li> The Kotlin standard library and out-of-the-box features</li> Writing clean and idiomatic code</li> Combining Kotlin and Java</li> Improve code reliability with null safety</li> Domain-specific languages</li> Kotlin coroutines and flows</li> Mastering the kotlinx.coroutines library</li> </ul> Kotlin in Action, Second Edition</i> is a complete guide to the Kotlin language written especially for readers familiar with Java or another OO language. Its authors—all core Kotlin language developers and Kotlin team members—share their unique insights, along with practical techniques and hands-on examples. This new second edition is fully updated to include the latest innovations, and it adds new chapters dedicated to coroutines, flows, and concurrency.
- 105APKUpdater is an open source tool that simplifies the process of finding updates for your installed apps.
https://github.com/rumboalla/apkupdater
APKUpdater is an open source tool that simplifies the process of finding updates for your installed apps. - rumboalla/apkupdater
- 106An open-source auto clicker on images for Android
https://github.com/Nain57/Smart-AutoClicker
An open-source auto clicker on images for Android. Contribute to Nain57/Smart-AutoClicker development by creating an account on GitHub.
- 107Model Forge is a library to automate model generation for automated testing
https://github.com/HelloCuriosity/model-forge
Model Forge is a library to automate model generation for automated testing - HelloCuriosity/model-forge
- 108kotlin multiplatform implementation/bindings of opentest4j
https://github.com/willowtreeapps/opentest4k
kotlin multiplatform implementation/bindings of opentest4j - willowtreeapps/opentest4k
- 109Library support for Kotlin coroutines
https://github.com/Kotlin/kotlinx.coroutines
Library support for Kotlin coroutines . Contribute to Kotlin/kotlinx.coroutines development by creating an account on GitHub.
- 110Android Mobile app to rule all Flipper's family
https://github.com/flipperdevices/Flipper-Android-App
Android Mobile app to rule all Flipper's family. Contribute to flipperdevices/Flipper-Android-App development by creating an account on GitHub.
- 111Official Jetpack Compose samples.
https://github.com/android/compose-samples
Official Jetpack Compose samples. Contribute to android/compose-samples development by creating an account on GitHub.
- 112A JMock wrapper for Kotlin
https://github.com/dmcg/k-sera
A JMock wrapper for Kotlin. Contribute to dmcg/k-sera development by creating an account on GitHub.
- 113Build Asynchronous Servers and Clients in Kotlin
https://ktor.io/
Kotlin Server and Client Framework for microservices, HTTP APIs, and RESTful services
- 114Search | Packt Subscription
https://www.packtpub.com/application-development/reactive-programming-kotlin
Search over 7,500 Programming & Development eBooks and videos to advance your IT skills, including Web Development, Application Development and Networking
- 115Search | Packt Subscription
https://www.packtpub.com/application-development/android-development-kotlin
Search over 7,500 Programming & Development eBooks and videos to advance your IT skills, including Web Development, Application Development and Networking
- 116Search | Packt Subscription
https://www.packtpub.com/application-development/mastering-android-development-kotlin
Search over 7,500 Programming & Development eBooks and videos to advance your IT skills, including Web Development, Application Development and Networking
- 117Universal Pasteboard Across Devices
https://github.com/CrossPaste/crosspaste-desktop
Universal Pasteboard Across Devices. Contribute to CrossPaste/crosspaste-desktop development by creating an account on GitHub.
- 118Generate unit testing boilerplate from kotlin files.
https://github.com/EranBoudjnah/TestIt
Generate unit testing boilerplate from kotlin files. - EranBoudjnah/TestIt
- 119BDD assertion library for Kotlin
https://github.com/winterbe/expekt
BDD assertion library for Kotlin. Contribute to winterbe/expekt development by creating an account on GitHub.
- 120Effective Kotlin
https://kt.academy/book/effectivekotlin
A book dedicated for Kotlin developers, helping to become better by writing safer, cleaner and more efficient code.
- 121Getting Started
https://www.pluralsight.com/courses/building-android-apps-kotlin-getting-started
Dive into the language set to replace Java while building Android applications. This course will give you a foundation of both Kotlin and Android skills to allow you to build apps faster and cleaner than ever before. Start learning by taking this beginners course from Pluralsight today!
- 122Ockero: Kotlin OpenGl Game Engine
https://github.com/KogeLabs/Ockero
Ockero: Kotlin OpenGl Game Engine. Contribute to KogeLabs/Ockero development by creating an account on GitHub.
- 123GraphQL toolkit for Kotlin.
https://github.com/AurityLab/graphql-kotlin-toolkit
GraphQL toolkit for Kotlin. Contribute to AurityLab/graphql-kotlin-toolkit development by creating an account on GitHub.
- 124The Official Conference App for DroidKaigi 2024
https://github.com/DroidKaigi/conference-app-2024
The Official Conference App for DroidKaigi 2024. Contribute to DroidKaigi/conference-app-2024 development by creating an account on GitHub.
- 125Static code analysis for Kotlin
https://github.com/detekt/detekt
Static code analysis for Kotlin. Contribute to detekt/detekt development by creating an account on GitHub.
- 126Testing tools for kotlinc and kapt
https://github.com/permissions-dispatcher/kompile-testing
Testing tools for kotlinc and kapt. Contribute to permissions-dispatcher/kompile-testing development by creating an account on GitHub.
- 127Opensource automated insulin delivery system (closed loop)
https://github.com/nightscout/AndroidAPS
Opensource automated insulin delivery system (closed loop) - nightscout/AndroidAPS
- 128provides metadata for chains
https://github.com/ethereum-lists/chains
provides metadata for chains. Contribute to ethereum-lists/chains development by creating an account on GitHub.
- 129Zeko SQL Builder is a high-performance lightweight SQL query library written for Kotlin language
https://github.com/darkredz/Zeko-SQL-Builder
Zeko SQL Builder is a high-performance lightweight SQL query library written for Kotlin language - darkredz/Zeko-SQL-Builder
- 130Kotlinwind CSS
https://github.com/allangomes/kotlinwind.css
Kotlinwind CSS. Contribute to allangomes/kotlinwind.css development by creating an account on GitHub.
- 131Asynchronous web framework for Kotlin. Create REST APIs in Kotlin easily with automatic Swagger/OpenAPI doc generation
https://github.com/darkredz/zeko-restapi-framework
Asynchronous web framework for Kotlin. Create REST APIs in Kotlin easily with automatic Swagger/OpenAPI doc generation - darkredz/zeko-restapi-framework
- 132The modular web framework for Java and Kotlin
https://github.com/jooby-project/jooby
The modular web framework for Java and Kotlin. Contribute to jooby-project/jooby development by creating an account on GitHub.
- 133A simple, rational music player for android
https://github.com/OxygenCobalt/Auxio
A simple, rational music player for android. Contribute to OxygenCobalt/Auxio development by creating an account on GitHub.
- 134A collection of samples to discuss and showcase different architectural tools and patterns for Android apps.
https://github.com/android/architecture-samples
A collection of samples to discuss and showcase different architectural tools and patterns for Android apps. - android/architecture-samples
- 135From Java to Kotlin Cheat Sheet
https://github.com/fabiomsr/from-java-to-kotlin
From Java to Kotlin Cheat Sheet. Contribute to fabiomsr/from-java-to-kotlin development by creating an account on GitHub.
- 136Modern Reactive CQRS Architecture Microservice development framework based on DDD and EventSourcing | 基于 DDD & EventSourcing 的现代响应式 CQRS 架构微服务开发框架
https://github.com/Ahoo-Wang/Wow
Modern Reactive CQRS Architecture Microservice development framework based on DDD and EventSourcing | 基于 DDD & EventSourcing 的现代响应式 CQRS 架构微服务开发框架 - Ahoo-Wang/Wow
- 137Easily build reactive web-apps in Kotlin based on flows and coroutines.
https://github.com/jwstegemann/fritz2
Easily build reactive web-apps in Kotlin based on flows and coroutines. - jwstegemann/fritz2
- 138SpringMockK: MockBean and SpyBean, but for MockK instead of Mockito
https://github.com/Ninja-Squad/springmockk
SpringMockK: MockBean and SpyBean, but for MockK instead of Mockito - Ninja-Squad/springmockk
- 139Tivi is a TV show tracking Android app, which connects to trakt.tv
https://github.com/chrisbanes/tivi
Tivi is a TV show tracking Android app, which connects to trakt.tv - chrisbanes/tivi
- 140DataBackup for Android 7.0+
https://github.com/XayahSuSuSu/Android-DataBackup
DataBackup for Android 7.0+. Contribute to XayahSuSuSu/Android-DataBackup development by creating an account on GitHub.
- 141Selfie JVM Snapshot Testing
https://selfie.dev/jvm
Zero-config inline and disk snapshots for Java, Kotlin, and more. Features garbage collection, filesystem-like APIs for snapshot data, and novel techniques for storytelling within test code.
- 142🖼️ Image Toolbox is an powerful picture editor, which can crop, apply filters, add some drawing, erase background, edit EXIF or even create PDF file
https://github.com/T8RIN/ImageToolbox
🖼️ Image Toolbox is an powerful picture editor, which can crop, apply filters, add some drawing, erase background, edit EXIF or even create PDF file - T8RIN/ImageToolbox
- 143A collection of samples of different Android OS platform APIs.
https://github.com/android/platform-samples
A collection of samples of different Android OS platform APIs. - android/platform-samples
- 144An assertion library for Kotlin
https://github.com/robfletcher/strikt
An assertion library for Kotlin. Contribute to robfletcher/strikt development by creating an account on GitHub.
- 145KoMock - Simple HTTP/Consul/SpringConfig http server framework written in Kotlin. Wiremock use cases
https://github.com/laviua/komock
KoMock - Simple HTTP/Consul/SpringConfig http server framework written in Kotlin. Wiremock use cases - laviua/komock
- 146A utility to make Kotlin/Java tests random yet reproducible
https://github.com/xgouchet/Elmyr
A utility to make Kotlin/Java tests random yet reproducible - xgouchet/Elmyr
- 147Joda Time and Java 8 Time Extensions for Kotlin
https://github.com/debop/koda-time
Joda Time and Java 8 Time Extensions for Kotlin. Contribute to debop/koda-time development by creating an account on GitHub.
- 148Kotlin in Action
https://manning.com/books/kotlin-in-action
Kotlin in Action</i> guides experienced Java developers from the language basics of Kotlin all the way through building applications to run on the JVM and Android devices.</p>
- 149基于无障碍,高级选择器,订阅规则的自定义屏幕点击 Android 应用 | An Android APP with custom screen tapping based on Accessibility, Advanced Selectors, and Subscription Rules
https://github.com/gkd-kit/gkd
基于无障碍,高级选择器,订阅规则的自定义屏幕点击 Android 应用 | An Android APP with custom screen tapping based on Accessibility, Advanced Selectors, and Subscription Rules - gkd-kit/gkd
- 150Media server for comics/mangas/BDs/magazines/eBooks with API, OPDS and Kobo Sync support
https://github.com/gotson/komga
Media server for comics/mangas/BDs/magazines/eBooks with API, OPDS and Kobo Sync support - gotson/komga
- 151Kotlin Evolution and Enhancement Process
https://github.com/Kotlin/KEEP
Kotlin Evolution and Enhancement Process. Contribute to Kotlin/KEEP development by creating an account on GitHub.
- 152assertions for kotlin inspired by assertj
https://github.com/willowtreeapps/assertk
assertions for kotlin inspired by assertj. Contribute to willowtreeapps/assertk development by creating an account on GitHub.
- 153Writing full-stack statically-typed web apps on JVM at its simplest
https://github.com/mvysny/vaadin-on-kotlin
Writing full-stack statically-typed web apps on JVM at its simplest - mvysny/vaadin-on-kotlin
- 154Hamcrest for Kotlin
https://github.com/npryce/hamkrest
Hamcrest for Kotlin. Contribute to npryce/hamkrest development by creating an account on GitHub.
- 155Kotlin Serverless Framework
https://github.com/jetbrains/kotless
Kotlin Serverless Framework. Contribute to JetBrains/kotless development by creating an account on GitHub.
- 156Kotlin multiplatform benchmarking toolkit
https://github.com/Kotlin/kotlinx-benchmark
Kotlin multiplatform benchmarking toolkit. Contribute to Kotlin/kotlinx-benchmark development by creating an account on GitHub.
- 157Kotlin/Java library and cli tool for scraping posts and media from various sources with neither authorization nor full page rendering (Facebook, Instagram, Twitter, Youtube, Tiktok, Telegram, Twitch, Reddit, 9GAG, Pinterest, Flickr, Tumblr, Coub, Vimeo, IFunny, VK, Odnoklassniki, Pikabu)
https://github.com/sokomishalov/skraper
Kotlin/Java library and cli tool for scraping posts and media from various sources with neither authorization nor full page rendering (Facebook, Instagram, Twitter, Youtube, Tiktok, Telegram, Twitc...
- 158Validation library. Fluent syntax in Java, mini DSL in Kotlin
https://github.com/deva666/KValidation
Validation library. Fluent syntax in Java, mini DSL in Kotlin - deva666/KValidation
- 159Kotlin plugin for Vim. Featuring: syntax highlighting, basic indentation, Syntastic support
https://github.com/udalov/kotlin-vim
Kotlin plugin for Vim. Featuring: syntax highlighting, basic indentation, Syntastic support - udalov/kotlin-vim
- 160Port of a popular ruby faker gem written in kotlin. Generate realistically looking fake data such as names, addresses, banking details, and many more, that can be used for testing and data anonymization purposes.
https://github.com/serpro69/kotlin-faker
Port of a popular ruby faker gem written in kotlin. Generate realistically looking fake data such as names, addresses, banking details, and many more, that can be used for testing and data anonymiz...
- 161A truly hackable editor: simple, lightweight, understandable
https://github.com/ftomassetti/kanvas
A truly hackable editor: simple, lightweight, understandable - ftomassetti/kanvas
- 162Repository for learning Kotlin Flow API
https://github.com/rozkminiacz/FlowRiddles
Repository for learning Kotlin Flow API. Contribute to rozkminiacz/FlowRiddles development by creating an account on GitHub.
- 163Lenses for Kotlin
https://github.com/poetix/klenses
Lenses for Kotlin. Contribute to poetix/klenses development by creating an account on GitHub.
- 164Fluent assertions for Kotlin
https://github.com/from-source/kiwi
Fluent assertions for Kotlin. Contribute to from-source/kiwi development by creating an account on GitHub.
- 165Monads for Kotlin
https://github.com/h0tk3y/kotlin-monads
Monads for Kotlin. Contribute to h0tk3y/kotlin-monads development by creating an account on GitHub.
- 166A simple Kotlin library to Query over Json Data.
https://github.com/s4kibs4mi/kotlin-jsonq
A simple Kotlin library to Query over Json Data. Contribute to s4kibs4mi/kotlin-jsonq development by creating an account on GitHub.
- 167Adds syntax highlighting to Kotlin files in Atom
https://github.com/alexmt/atom-kotlin-language
Adds syntax highlighting to Kotlin files in Atom. Contribute to alexmt/atom-kotlin-language development by creating an account on GitHub.
- 168RBAC-based And Policy-based Multi-Tenant Reactive Security Framework | 基于 RBAC 和策略的多租户响应式安全框架
https://github.com/Ahoo-Wang/CoSec
RBAC-based And Policy-based Multi-Tenant Reactive Security Framework | 基于 RBAC 和策略的多租户响应式安全框架 - Ahoo-Wang/CoSec
- 169A multiplatform WebGPU 2D game framework written in Kotlin. Build your own game engine on top.
https://github.com/littlektframework/littlekt
A multiplatform WebGPU 2D game framework written in Kotlin. Build your own game engine on top. - littlektframework/littlekt
- 170Kotlin language support for VS Code
https://github.com/mathiasfrohlich/vscode-kotlin
Kotlin language support for VS Code. Contribute to mathiasfrohlich/vscode-kotlin development by creating an account on GitHub.
- 171Object notation in pure Kotlin!
https://github.com/Jire/KTON
Object notation in pure Kotlin! Contribute to Jire/KTON development by creating an account on GitHub.
- 172Gson TypeAdapter & Factory generator for Kotlin data classes
https://github.com/aafanasev/kson
Gson TypeAdapter & Factory generator for Kotlin data classes - aafanasev/kson
- 173A fast, Kotlin-native Markdown parser
https://github.com/nickhristov/krakdown
A fast, Kotlin-native Markdown parser. Contribute to nickhristov/krakdown development by creating an account on GitHub.
- 174A private messenger for Android.
https://github.com/signalapp/Signal-Android
A private messenger for Android. Contribute to signalapp/Signal-Android development by creating an account on GitHub.
- 175A library for testing Kotlin and Java annotation processors, compiler plugins and code generation
https://github.com/tschuchortdev/kotlin-compile-testing
A library for testing Kotlin and Java annotation processors, compiler plugins and code generation - tschuchortdev/kotlin-compile-testing
- 176A small wrapper for the Kotlin compiler that can be used to execute .kts scripts
https://github.com/cypressious/KotlinW
A small wrapper for the Kotlin compiler that can be used to execute .kts scripts - cypressious/KotlinW
- 177A Kernel based root solution for Android
https://github.com/tiann/KernelSU
A Kernel based root solution for Android. Contribute to tiann/KernelSU development by creating an account on GitHub.
- 178Design Patterns implemented in Kotlin
https://github.com/dbacinski/Design-Patterns-In-Kotlin
Design Patterns implemented in Kotlin. Contribute to dbacinski/Design-Patterns-In-Kotlin development by creating an account on GitHub.
- 179Detection of design principle violations in Kotlin as a plugin to detekt.
https://github.com/mkohm/detekt-hint
Detection of design principle violations in Kotlin as a plugin to detekt. - Mkohm/detekt-hint
- 180URLSome - URL builder library for Kotlin
https://github.com/mobiletoly/urlsome
URLSome - URL builder library for Kotlin. Contribute to mobiletoly/urlsome development by creating an account on GitHub.
- 181Spring Data with Requery
https://github.com/coupang/spring-data-requery
Spring Data with Requery. Contribute to coupang/spring-data-requery development by creating an account on GitHub.
- 182Kotlin coroutine support for MongoDB built on top of the official Reactive Streams Java Driver
https://github.com/fluidsonic/fluid-mongo
Kotlin coroutine support for MongoDB built on top of the official Reactive Streams Java Driver - fluidsonic/fluid-mongo
- 183MapDB provides concurrent Maps, Sets and Queues backed by disk storage or off-heap-memory. It is a fast and easy to use embedded Java database engine.
https://github.com/jankotek/mapdb
MapDB provides concurrent Maps, Sets and Queues backed by disk storage or off-heap-memory. It is a fast and easy to use embedded Java database engine. - jankotek/mapdb
- 184Thelema - 3D graphics engine, written in Kotlin. Based on sources of libGDX.
https://github.com/zeganstyl/thelema-engine
Thelema - 3D graphics engine, written in Kotlin. Based on sources of libGDX. - zeganstyl/thelema-engine
- 185Type-safe dimensional analysis and unit conversion in Kotlin.
https://github.com/kunalsheth/units-of-measure
Type-safe dimensional analysis and unit conversion in Kotlin. - kunalsheth/units-of-measure
- 186simondankelmann/Bluetooth-LE-Spam
https://github.com/simondankelmann/Bluetooth-LE-Spam
Contribute to simondankelmann/Bluetooth-LE-Spam development by creating an account on GitHub.
- 187Simplest & Powerful Testing Framework For Kotlin. No annotations!
https://github.com/mvysny/DynaTest
Simplest & Powerful Testing Framework For Kotlin. No annotations! - mvysny/dynatest
- 188🇧🇷 Validador simples para o documento CPF (Cadastro de Pessoa Física) | Simple Brazilian taxpayer document (CPF) validator
https://github.com/LeoColman/SimpleCpfValidator
🇧🇷 Validador simples para o documento CPF (Cadastro de Pessoa Física) | Simple Brazilian taxpayer document (CPF) validator - LeoColman/SimpleCpfValidator
- 189Android Database - first and fast, lightweight on-device vector database
https://github.com/objectbox/objectbox-java
Android Database - first and fast, lightweight on-device vector database - objectbox/objectbox-java
- 190Kotlin wrapper of mbedtls also for java
https://github.com/open-coap/kotlin-mbedtls
Kotlin wrapper of mbedtls also for java. Contribute to open-coap/kotlin-mbedtls development by creating an account on GitHub.
- 191A "batteries included" port of Reduxjs for Kotlin+Android
https://github.com/beyondeye/Reduks
A "batteries included" port of Reduxjs for Kotlin+Android - beyondeye/Reduks
- 192Kotlin Language Interactive Shell
https://github.com/Kotlin/kotlin-interactive-shell
Kotlin Language Interactive Shell. Contribute to Kotlin/kotlin-interactive-shell development by creating an account on GitHub.
- 193Library for executing .kts files from regular Kotlin code
https://github.com/s1monw1/KtsRunner
Library for executing .kts files from regular Kotlin code - s1monw1/KtsRunner
- 194LevelDB client for Kotlin and/or Java 8+
https://github.com/shyiko/levelkt
LevelDB client for Kotlin and/or Java 8+. Contribute to shyiko/levelkt development by creating an account on GitHub.
- 195Secure Kotlin scripting and binary lambda-scripts
https://github.com/kohesive/keplin
Secure Kotlin scripting and binary lambda-scripts. Contribute to kohesive/keplin development by creating an account on GitHub.
- 196Sublime Text 2 Package for Kotlin Programming Language
https://github.com/vkostyukov/kotlin-sublime-package
Sublime Text 2 Package for Kotlin Programming Language - vkostyukov/kotlin-sublime-package
- 197Percentage calculations made easy
https://github.com/eriksencosta/math-percentage
Percentage calculations made easy. Contribute to eriksencosta/math-percentage development by creating an account on GitHub.
- 198IBM Q Experience Kotlin toolkit - Kotlin library to interact and write assembly code for IBM Quantum computers
https://github.com/ssuukk/Qotlin
IBM Q Experience Kotlin toolkit - Kotlin library to interact and write assembly code for IBM Quantum computers - ssuukk/Qotlin
- 199Progress for Kotlin
https://github.com/mplatvoet/progress
Progress for Kotlin. Contribute to mplatvoet/progress development by creating an account on GitHub.
- 200Authoring GitHub Actions workflows in Kotlin. You won't go back to YAML!
https://github.com/typesafegithub/github-workflows-kt
Authoring GitHub Actions workflows in Kotlin. You won't go back to YAML! - typesafegithub/github-workflows-kt
- 201RxJava bindings for Kotlin
https://github.com/ReactiveX/RxKotlin
RxJava bindings for Kotlin. Contribute to ReactiveX/RxKotlin development by creating an account on GitHub.
- 202Querylight is an in memory, kotlin multi platform text indexing library that implements tf/idf and a minimal query language. Great for client side search in web apps, android or other Kotlin apps.
https://github.com/jillesvangurp/querylight
Querylight is an in memory, kotlin multi platform text indexing library that implements tf/idf and a minimal query language. Great for client side search in web apps, android or other Kotlin apps. ...
- 203A handy Database access library in Kotlin
https://github.com/seratch/kotliquery
A handy Database access library in Kotlin. Contribute to seratch/kotliquery development by creating an account on GitHub.
- 204Math conventions to reduce boilerplate code
https://github.com/eriksencosta/math-common
Math conventions to reduce boilerplate code. Contribute to eriksencosta/math-common development by creating an account on GitHub.
- 205Multiplatform kotlin string case conversion and detection library.
https://github.com/dotCipher/kase-format
Multiplatform kotlin string case conversion and detection library. - dotCipher/kase-format
- 206Minimalistic and multiplatform logging for Kotlin
https://github.com/Lewik/klog
Minimalistic and multiplatform logging for Kotlin. Contribute to Lewik/klog development by creating an account on GitHub.
- 207The Kotlin Programming Language.
https://github.com/JetBrains/kotlin
The Kotlin Programming Language. . Contribute to JetBrains/kotlin development by creating an account on GitHub.
- 208forkhandles/tuples4k at trunk · fork-handles/forkhandles
https://github.com/fork-handles/forkhandles/tree/trunk/tuples4k
Foundational libraries for Kotlin. Contribute to fork-handles/forkhandles development by creating an account on GitHub.
- 209Kotlin extensions for Java 8 java.time API
https://github.com/yole/kxdate
Kotlin extensions for Java 8 java.time API. Contribute to yole/kxdate development by creating an account on GitHub.
- 210SonarLint for IntelliJ
https://github.com/SonarSource/sonarlint-intellij
SonarLint for IntelliJ. Contribute to SonarSource/sonarlint-intellij development by creating an account on GitHub.
- 211Portable validations for Kotlin
https://github.com/konform-kt/konform
Portable validations for Kotlin. Contribute to konform-kt/konform development by creating an account on GitHub.
- 212The easiest HTTP networking library for Kotlin/Android
https://github.com/kittinunf/Fuel
The easiest HTTP networking library for Kotlin/Android - kittinunf/fuel
- 213A concise and lightweight Kotlin DSL to build JSON objects and render their String representations
https://github.com/lectra-tech/koson
A concise and lightweight Kotlin DSL to build JSON objects and render their String representations - lectra-tech/koson
- 214🐫🐍🍢🅿 Multiplatform Kotlin library to convert strings between various case formats including Camel Case, Snake Case, Pascal Case and Kebab Case
https://github.com/pearxteam/kasechange
🐫🐍🍢🅿 Multiplatform Kotlin library to convert strings between various case formats including Camel Case, Snake Case, Pascal Case and Kebab Case - pearxteam/kasechange
- 215KMMBridge is a tool that helps publish Kotlin Multiplatform (KMP) Xcode binaries for use from Swift Package Manager (SPM) and CocoaPods.
https://github.com/touchlab/KMMBridge
KMMBridge is a tool that helps publish Kotlin Multiplatform (KMP) Xcode binaries for use from Swift Package Manager (SPM) and CocoaPods. - touchlab/KMMBridge
- 216A lightweight ORM framework for Kotlin with strong-typed SQL DSL and sequence APIs.
https://github.com/kotlin-orm/ktorm
A lightweight ORM framework for Kotlin with strong-typed SQL DSL and sequence APIs. - kotlin-orm/ktorm
- 217Build software better, together
https://github.com/trending?l=kotlin
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 218Ebean ORM
https://github.com/ebean-orm/ebean
Ebean ORM. Contribute to ebean-orm/ebean development by creating an account on GitHub.
- 219API documentation engine for Kotlin
https://github.com/Kotlin/dokka
API documentation engine for Kotlin. Contribute to Kotlin/dokka development by creating an account on GitHub.
- 220A high-performance Kotlin Native database driver for PostgreSQL, MySQL, and SQLite.
https://github.com/smyrgeorge/sqlx4k
A high-performance Kotlin Native database driver for PostgreSQL, MySQL, and SQLite. - smyrgeorge/sqlx4k
- 221This library provides a fluent DSL for querying spring data JPA repositories using spring data Specifications (i.e. the JPA Criteria API), without boilerplate code or a generated metamodel.
https://github.com/consoleau/kotlin-jpa-specification-dsl
This library provides a fluent DSL for querying spring data JPA repositories using spring data Specifications (i.e. the JPA Criteria API), without boilerplate code or a generated metamodel. - conso...
- 222Language extension for notepad++
https://github.com/ice1000/NppExtension
:smiley: Language extension for notepad++. Contribute to ice1000/NppExtension development by creating an account on GitHub.
- 223Kotlin based JHipster
https://github.com/jhipster/jhipster-kotlin
Kotlin based JHipster. Contribute to jhipster/jhipster-kotlin development by creating an account on GitHub.
- 224Kotlin DSL for Pi4J V2
https://github.com/Pi4J/pi4j-kotlin
Kotlin DSL for Pi4J V2. Contribute to Pi4J/pi4j-kotlin development by creating an account on GitHub.
- 225Functional companion to Kotlin's Compiler
https://github.com/arrow-kt/arrow-meta
Functional companion to Kotlin's Compiler. Contribute to arrow-kt/arrow-meta development by creating an account on GitHub.
- 226Module that adds support for serialization/deserialization of Kotlin (http://kotlinlang.org) classes and data classes.
https://github.com/FasterXML/jackson-module-kotlin
Module that adds support for serialization/deserialization of Kotlin (http://kotlinlang.org) classes and data classes. - FasterXML/jackson-module-kotlin
- 227NoSQL database query and access library for Kotlin
https://github.com/cheptsov/kotlin-nosql
NoSQL database query and access library for Kotlin - cheptsov/kotlin-nosql
- 228requery - modern SQL based query & persistence for Java / Kotlin / Android
https://github.com/requery/requery
requery - modern SQL based query & persistence for Java / Kotlin / Android - requery/requery
- 229Idiomatic persistence layer for Kotlin
https://github.com/TouK/krush
Idiomatic persistence layer for Kotlin. Contribute to TouK/krush development by creating an account on GitHub.
- 230An Object Graph Mapping Library for Kotlin and Gremlin
https://github.com/pm-dev/kotlin-gremlin-ogm
An Object Graph Mapping Library for Kotlin and Gremlin - pm-dev/kotlin-gremlin-ogm
- 231Do comprehensions for Kotlin and 3rd party libraries [STABLE]
https://github.com/pakoito/Komprehensions
Do comprehensions for Kotlin and 3rd party libraries [STABLE] - pakoito/Komprehensions
- 232Easy PDF generation with HTML & CSS using Chromium or Google Chrome
https://github.com/fluidsonic/fluid-pdf
Easy PDF generation with HTML & CSS using Chromium or Google Chrome - fluidsonic/fluid-pdf
- 233Lightweight library allowing to introspect basic stuff about Kotlin symbols
https://github.com/Kotlin/kotlinx.reflect.lite
Lightweight library allowing to introspect basic stuff about Kotlin symbols - Kotlin/kotlinx.reflect.lite
- 234Port of Adobe's XMP SDK for Kotlin Multiplatform
https://github.com/Ashampoo/xmpcore
Port of Adobe's XMP SDK for Kotlin Multiplatform. Contribute to Ashampoo/xmpcore development by creating an account on GitHub.
- 235kotlin typesafe builder for xpath
https://github.com/DetachHead/kotlinxpath
kotlin typesafe builder for xpath. Contribute to DetachHead/kotlinxpath development by creating an account on GitHub.
- 236🌶 A simple Kotlin web framework inspired by Clojure's Ring.
https://github.com/danneu/kog
🌶 A simple Kotlin web framework inspired by Clojure's Ring. - danneu/kog
- 237Textmate bundle for the Kotlin programming language
https://github.com/sargunster/kotlin-textmate-bundle
Textmate bundle for the Kotlin programming language - sargunv/kotlin-textmate-bundle
- 238Kotlin compiler plugin to hide secret data
https://github.com/aafanasev/sekret
Kotlin compiler plugin to hide secret data. Contribute to aafanasev/sekret development by creating an account on GitHub.
- 239Lightweight logging library for Kotlin/Multiplatform. Supports Android, iOS, JavaScript and plain JVM environments.
https://github.com/saschpe/log4k
Lightweight logging library for Kotlin/Multiplatform. Supports Android, iOS, JavaScript and plain JVM environments. - saschpe/Log4K
- 240A powerful in-process event dispatcher based on Kotlin and Coroutines.
https://github.com/RationalityFrontline/kevent
A powerful in-process event dispatcher based on Kotlin and Coroutines. - RationalityFrontline/KEvent
- 241Simple multiplatform logging in Kotlin
https://github.com/InsanusMokrassar/KSLog
Simple multiplatform logging in Kotlin. Contribute to InsanusMokrassar/KSLog development by creating an account on GitHub.
- 242Multiplaform kotlin library for calculating text differences. Based on java-diff-utils, supports JVM, JS and native targets.
https://github.com/petertrr/kotlin-multiplatform-diff
Multiplaform kotlin library for calculating text differences. Based on java-diff-utils, supports JVM, JS and native targets. - petertrr/kotlin-multiplatform-diff
- 243Java & Kotlin Async DataBase Driver for MySQL and PostgreSQL written in Kotlin
https://github.com/jasync-sql/jasync-sql
Java & Kotlin Async DataBase Driver for MySQL and PostgreSQL written in Kotlin - jasync-sql/jasync-sql
- 244🦄Intuitive and powerful human-readable Kotlin DSL for IPCs & turning anything into a message receiver / broadcaster🦄
https://github.com/DavidMellul/Kotlin-Publish-Subscribe
🦄Intuitive and powerful human-readable Kotlin DSL for IPCs & turning anything into a message receiver / broadcaster🦄 - DavidMellul/Kotlin-Publish-Subscribe
- 245基于Kotlin的整合代码框架,为标准库和其他框架提供各种有用的扩展。Integrated code framework based on Kotlin, provides many useful extensions for standard library and some frameworks.
https://github.com/DragonKnightOfBreeze/breeze-framework
基于Kotlin的整合代码框架,为标准库和其他框架提供各种有用的扩展。Integrated code framework based on Kotlin, provides many useful extensions for standard library and some frameworks. - DragonKnightOfBreeze/Breeze-Framework
- 246A modern MAVLink library for Kotlin Multiplatform.
https://github.com/divyanshupundir/mavlink-kotlin
A modern MAVLink library for Kotlin Multiplatform. - divyanshupundir/mavlink-kotlin
- 247Kovenant. Promises for Kotlin.
https://github.com/mplatvoet/kovenant
Kovenant. Promises for Kotlin. Contribute to mplatvoet/kovenant development by creating an account on GitHub.
- 248Kotlin wrapper for StAX
https://github.com/asm0dey/staks
Kotlin wrapper for StAX. Contribute to asm0dey/staks development by creating an account on GitHub.
- 249A multiplatform Result monad for modelling success or failure operations.
https://github.com/michaelbull/kotlin-result
A multiplatform Result monad for modelling success or failure operations. - michaelbull/kotlin-result
- 250KorGE Game Engine. Multiplatform Kotlin Game Engine
https://github.com/korlibs/KorGE
KorGE Game Engine. Multiplatform Kotlin Game Engine - korlibs/korge
- 251Gradle Script plugin to generate documentation by Dokka documentation engine in Javadoc or other formats for Java, Kotlin, Android and non-Android projects. It's very easy, you don't need to add to dependencies section additional classpath or think about compatibility issues, you don't need additional repositories also.
https://github.com/Vorlonsoft/EasyDokkaPlugin
Gradle Script plugin to generate documentation by Dokka documentation engine in Javadoc or other formats for Java, Kotlin, Android and non-Android projects. It's very easy, you don't need t...
- 252Kotliny Network is a simple, powerful and lightweight Kotlin Multiplatform Network Client.
https://github.com/corbella83/kotliny.network
Kotliny Network is a simple, powerful and lightweight Kotlin Multiplatform Network Client. - corbella83/kotliny.network
- 253A state container for Java & Kotlin, inspired by Redux & Elm
https://github.com/brianegan/bansa
A state container for Java & Kotlin, inspired by Redux & Elm - brianegan/bansa
- 254Zircon is an extensible and user-friendly, multiplatform tile engine.
https://github.com/Hexworks/zircon
Zircon is an extensible and user-friendly, multiplatform tile engine. - Hexworks/zircon
- 255A simple and modern Java and Kotlin vert.x web framework
https://github.com/cloudoptlab/cloudopt-next
A simple and modern Java and Kotlin vert.x web framework - cloudoptlab/cloudopt-next
- 256Transactional schema-less embedded database used by JetBrains YouTrack and JetBrains Hub.
https://github.com/JetBrains/xodus
Transactional schema-less embedded database used by JetBrains YouTrack and JetBrains Hub. - JetBrains/xodus
- 257forkhandles/parser4k at trunk · fork-handles/forkhandles
https://github.com/fork-handles/forkhandles/tree/trunk/parser4k
Foundational libraries for Kotlin. Contribute to fork-handles/forkhandles development by creating an account on GitHub.
- 258A Kotlin Multiplatform porting for MMKV.
https://github.com/ctripcorp/mmkv-kotlin
A Kotlin Multiplatform porting for MMKV. Contribute to ctripcorp/mmkv-kotlin development by creating an account on GitHub.
- 259Intuitive, type-safe units of measure
https://github.com/nacular/measured
Intuitive, type-safe units of measure. Contribute to nacular/measured development by creating an account on GitHub.
- 260Kotlin Cheat Sheet
https://blog.kotlin-academy.com/kotlin-cheat-sheet-1137588c75a
We prepared for you Kotlin Cheat sheet, so you can have the most important elements close at hand!
- 261The invisible REST and web framework
https://github.com/kohesive/kovert
The invisible REST and web framework. Contribute to kohesive/kovert development by creating an account on GitHub.
- 262[deprecated] KMongo - a Kotlin toolkit for Mongo
https://github.com/Litote/kmongo
[deprecated] KMongo - a Kotlin toolkit for Mongo. Contribute to Litote/kmongo development by creating an account on GitHub.
- 263The official MongoDB drivers for Java, Kotlin, and Scala
https://github.com/mongodb/mongo-java-driver
The official MongoDB drivers for Java, Kotlin, and Scala - mongodb/mongo-java-driver
- 264Simple, Expressive, Extensible Testing for Kotlin on the JVM
https://github.com/dmcg/minutest
Simple, Expressive, Extensible Testing for Kotlin on the JVM - dmcg/minutest
- 265Kotlin framework for conversational voice assistants and chatbots development
https://github.com/just-ai/jaicf-kotlin
Kotlin framework for conversational voice assistants and chatbots development - just-ai/jaicf-kotlin
- 266Kotlin Symbol Processing API
https://github.com/google/ksp
Kotlin Symbol Processing API. Contribute to google/ksp development by creating an account on GitHub.
- 267Kotlin/kotlinx-kover
https://github.com/Kotlin/kotlinx-kover
Contribute to Kotlin/kotlinx-kover development by creating an account on GitHub.
- 268The modelling for success/failure of operations in Kotlin and KMM (Kotlin Multiplatform Mobile)
https://github.com/kittinunf/Result
The modelling for success/failure of operations in Kotlin and KMM (Kotlin Multiplatform Mobile) - kittinunf/Result
- 269Kotlin DSL for easier RxJava testing.
https://github.com/RubyLichtenstein/RxTest
Kotlin DSL for easier RxJava testing. Contribute to RubyLichtenstein/RxTest development by creating an account on GitHub.
- 270validator for kotlin json serialization
https://github.com/marifeta/kvalidator
validator for kotlin json serialization. Contribute to Marifeta/kvalidator development by creating an account on GitHub.
- 271Learning Programming With Kotlin
https://www.udemy.com/kotlin-course/
Learn Kotlin from scratch! Grasp object-orientation and idiomatic Kotlin to realize coding projects and Android apps!
- 272A JavaScript Object Notation library for Kotlin JVM.
https://github.com/Shengaero/kotlin-json
A JavaScript Object Notation library for Kotlin JVM. - Shengaero/kotlin-json
- 273⚠️ Stop saying "you forgot to …" in code review
https://github.com/danger/kotlin
⚠️ Stop saying "you forgot to …" in code review. Contribute to danger/kotlin development by creating an account on GitHub.
- 274Helper to upload Gradle Android Artifacts, Gradle Java Artifacts and Gradle Kotlin Artifacts to Maven repositories (JCenter, Maven Central, Corporate staging/snapshot servers and local Maven repositories).
https://github.com/Vorlonsoft/GradleMavenPush
Helper to upload Gradle Android Artifacts, Gradle Java Artifacts and Gradle Kotlin Artifacts to Maven repositories (JCenter, Maven Central, Corporate staging/snapshot servers and local Maven reposi...
- 275Image metadata manipulation library for Kotlin Multiplatform
https://github.com/Ashampoo/kim
Image metadata manipulation library for Kotlin Multiplatform - Ashampoo/kim
- 276A REST request routing layer for AWS lambda handlers written in Kotlin
https://github.com/moia-dev/lambda-kotlin-request-router
A REST request routing layer for AWS lambda handlers written in Kotlin - GitHub - moia-oss/lambda-kotlin-request-router: A REST request routing layer for AWS lambda handlers written in Kotlin
- 277Λrrow - Functional companion to Kotlin's Standard Library
https://github.com/arrow-kt/arrow
Λrrow - Functional companion to Kotlin's Standard Library - arrow-kt/arrow
- 278A Kotlin/Java library for intelligent object mapping and conversion between objects.
https://github.com/krud-dev/shapeshift
A Kotlin/Java library for intelligent object mapping and conversion between objects. - krud-dev/shapeshift
- 279A program that reformats Kotlin source code to comply with the common community standard for Kotlin code conventions.
https://github.com/facebookincubator/ktfmt
A program that reformats Kotlin source code to comply with the common community standard for Kotlin code conventions. - facebook/ktfmt
- 280Ksoup is a Kotlin Multiplatform library for working with HTML and XML. It's a port of the renowned Java library Jsoup.
https://github.com/fleeksoft/ksoup
Ksoup is a Kotlin Multiplatform library for working with HTML and XML. It's a port of the renowned Java library Jsoup. - fleeksoft/ksoup
- 281Telegram Bot API wrapper with handy Kotlin DSL.
https://github.com/vendelieu/telegram-bot
Telegram Bot API wrapper with handy Kotlin DSL. Contribute to vendelieu/telegram-bot development by creating an account on GitHub.
- 282An anti-bikeshedding Kotlin linter with built-in formatter
https://github.com/pinterest/ktlint
An anti-bikeshedding Kotlin linter with built-in formatter - pinterest/ktlint
- 283Logging DSL implementation in Kotlin
https://github.com/d-max/dsl-logger
Logging DSL implementation in Kotlin. Contribute to dybarsky/dsl-logger development by creating an account on GitHub.
- 284Powerful, elegant and flexible test framework for Kotlin with assertions, property testing and data driven tests.
https://github.com/kotest/kotest
Powerful, elegant and flexible test framework for Kotlin with assertions, property testing and data driven tests. - kotest/kotest
- 285Getting Started
https://www.raywenderlich.com/7109-test-driven-development-tutorial-for-android-getting-started
Learn the basics of test-driven development, or TDD, and discover how to use TDD effectively when developing your Android apps!
- 286RxJava2 wrapper for aerospike-client-java
https://github.com/Ganet/rxaerospike
RxJava2 wrapper for aerospike-client-java. Contribute to jacinpoz/rxaerospike development by creating an account on GitHub.
- 287A JSON parser for Kotlin
https://github.com/cbeust/klaxon
A JSON parser for Kotlin. Contribute to cbeust/klaxon development by creating an account on GitHub.
- 288nitrite-java/potassium-nitrite at master · nitrite/nitrite-java
https://github.com/dizitart/nitrite-database/tree/master/potassium-nitrite
NoSQL embedded document store for Java. Contribute to nitrite/nitrite-java development by creating an account on GitHub.
- 289Kotlin 1.1 async clients for sync protocols: Mysql, Postgres, Thrift, Http
https://github.com/KotlinPorts/kt-postgresql-async
Kotlin 1.1 async clients for sync protocols: Mysql, Postgres, Thrift, Http - KotlinPorts/pooled-client
- 290A blazing fast, powerful, and very simple ORM android database library that writes database code for you.
https://github.com/Raizlabs/DBFlow
A blazing fast, powerful, and very simple ORM android database library that writes database code for you. - agrosner/DBFlow
- 291Fixture factory in Kotlin
https://github.com/bluegroundltd/kfactory
Fixture factory in Kotlin. Contribute to bluegroundltd/kfactory development by creating an account on GitHub.
- 292A Kotlin-based DSL for text adventures, with a partial replica of the classic Colossal Cave as an example.
https://github.com/vassilibykov/AdventKT
A Kotlin-based DSL for text adventures, with a partial replica of the classic Colossal Cave as an example. - ast-hugger/AdventKT
- 293Kt. Academy
https://blog.kotlin-academy.com/
Blog with mission to simplify Kotlin learning.
- 294Predictable state container for Kotlin apps
https://github.com/pardom/redux-kotlin
Predictable state container for Kotlin apps. Contribute to pardom-zz/redux-kotlin development by creating an account on GitHub.
- 295forkhandles/result4k at trunk · fork-handles/forkhandles
https://github.com/fork-handles/forkhandles/blob/trunk/result4k
Foundational libraries for Kotlin. Contribute to fork-handles/forkhandles development by creating an account on GitHub.
- 296GraphQL request string builder written in Kotlin
https://github.com/taskworld/kraph
GraphQL request string builder written in Kotlin. Contribute to VerachadW/kraph development by creating an account on GitHub.
- 297Life is too short to google for dependencies and versions
https://github.com/jmfayard/refreshVersions
Life is too short to google for dependencies and versions - Splitties/refreshVersions
- 298A named {{ tag }} template processor in kotlin.
https://github.com/L-Briand/TT
A named {{ tag }} template processor in kotlin. Contribute to L-Briand/TagTemplate development by creating an account on GitHub.
- 299Using Mockito with Kotlin
https://github.com/nhaarman/mockito-kotlin
Using Mockito with Kotlin. Contribute to mockito/mockito-kotlin development by creating an account on GitHub.
- 300Simple unit conversion library for Kotlin
https://github.com/sargunster/KtUnits
Simple unit conversion library for Kotlin. Contribute to sargunv/ktunits development by creating an account on GitHub.
- 301A specification framework for Kotlin
https://github.com/JetBrains/spek
A specification framework for Kotlin. Contribute to spekframework/spek development by creating an account on GitHub.
- 302Kotlin implementation of Groovy Truth
https://github.com/JoelW-S/groothy
Kotlin implementation of Groovy Truth. Contribute to jw-s/groothy development by creating an account on GitHub.
- 303SQLDelight - Generates typesafe Kotlin APIs from SQL
https://github.com/square/sqldelight
SQLDelight - Generates typesafe Kotlin APIs from SQL - cashapp/sqldelight
- 304Simple parser combinator library for Kotlin
https://github.com/sargunster/CakeParse
Simple parser combinator library for Kotlin. Contribute to sargunv/cakeparse development by creating an account on GitHub.
- 305Feature-rich, efficient, and highly customizable plugins for your Multiplatform Ktor Server or Client
https://github.com/Flaxoos/flax-ktor-plugins
Feature-rich, efficient, and highly customizable plugins for your Multiplatform Ktor Server or Client - Flaxoos/extra-ktor-plugins
- 306Snapshot Testing framework for Kotlin.
https://github.com/karumi/KotlinSnapshot
Snapshot Testing framework for Kotlin. Contribute to pedrovgs/KotlinSnapshot development by creating an account on GitHub.
- 307:rocket: A strongly-typed, caching GraphQL client for the JVM, Android, and Kotlin multiplatform.
https://github.com/apollographql/apollo-android
:rocket: A strongly-typed, caching GraphQL client for the JVM, Android, and Kotlin multiplatform. - apollographql/apollo-kotlin
- 308Language Savant. If your repository's language is being reported incorrectly, send us a pull request!
https://github.com/github/linguist
Language Savant. If your repository's language is being reported incorrectly, send us a pull request! - github-linguist/linguist
- 309Guice DSL extensions for Kotlin
https://github.com/authzee/kotlin-guice
Guice DSL extensions for Kotlin. Contribute to misfitlabsdev/kotlin-guice development by creating an account on GitHub.
- 310Libraries for running GraphQL in Kotlin
https://github.com/ExpediaDotCom/graphql-kotlin
Libraries for running GraphQL in Kotlin. Contribute to ExpediaGroup/graphql-kotlin development by creating an account on GitHub.
- 311Kotlin code completion, diagnostics and more for any editor/IDE using the Language Server Protocol
https://github.com/fwcd/KotlinLanguageServer
Kotlin code completion, diagnostics and more for any editor/IDE using the Language Server Protocol - fwcd/kotlin-language-server
- 312The Kotlin fake data generator library!
https://github.com/moove-it/fakeit
The Kotlin fake data generator library! Contribute to thisisqubika/fakeit development by creating an account on GitHub.
- 313A simple and modern Java and Kotlin web framework
https://github.com/tipsy/javalin
A simple and modern Java and Kotlin web framework. Contribute to javalin/javalin development by creating an account on GitHub.
- 314A pure Ruby code highlighter that is compatible with Pygments
https://github.com/jneen/rouge
A pure Ruby code highlighter that is compatible with Pygments - rouge-ruby/rouge
- 315tinylog is a lightweight logging framework for Java, Kotlin, Scala, and Android
https://github.com/pmwmedia/tinylog
tinylog is a lightweight logging framework for Java, Kotlin, Scala, and Android - tinylog-org/tinylog
- 316Koin - a pragmatic lightweight dependency injection framework for Kotlin & Kotlin Multiplatform
https://github.com/Ekito/koin
Koin - a pragmatic lightweight dependency injection framework for Kotlin & Kotlin Multiplatform - InsertKoinIO/koin
- 317Painless Kotlin Dependency Injection
https://github.com/Kodein-Framework/Kodein-DI
Painless Kotlin Dependency Injection. Contribute to kosi-libs/Kodein development by creating an account on GitHub.
- 318Programming Fundamentals in Kotlin
https://www.coursera.org/learn/meta-programming-fundamentals-kotlin
Offered by Meta. Practice and expand on the fundamentals of programming that are core to any language as well as the unique aspects of ... Enroll for free.
- 319Kotlin for Java Developers
https://www.coursera.org/learn/kotlin-for-java-developers
Offered by JetBrains. The Kotlin programming language is a modern language that gives you more power for your everyday tasks. Kotlin is ... Enroll for free.
- 320Pure Kotlin CSV Reader/Writer
https://github.com/doyaaaaaken/kotlin-csv
Pure Kotlin CSV Reader/Writer. Contribute to jsoizo/kotlin-csv development by creating an account on GitHub.
- 321Advanced Programming in Kotlin
https://www.coursera.org/learn/advanced-programming-in-kotlin
Offered by Meta. In this course, you will expand your Kotlin fluency by exploring the advanced concepts used by many Kotlin programmers. You ... Enroll for free.
- 322CS194A Android Development
https://www.youtube.com/playlist?list=PL7NYbSE8uaBDcLkbXsQADdvBnVbavonGn
Learn basic, foundational techniques for developing Android mobile applications and apply those toward building a single or multi page, networked Android app...
- 323An HTTP Framework
https://github.com/hhariri/wasabi
An HTTP Framework. Contribute to wasabifx/wasabi development by creating an account on GitHub.
- 324Type-safe library for work with Telegram Bot API
https://github.com/insanusmokrassar/TelegramBotAPI
Type-safe library for work with Telegram Bot API. Contribute to InsanusMokrassar/ktgbotapi development by creating an account on GitHub.
- 325Unofficial Actions on Google SDK for Kotlin and Java
https://github.com/TicketmasterMobileStudio/actions-on-google-kotlin
Unofficial Actions on Google SDK for Kotlin and Java - ticketmaster/actions-on-google-kotlin
- 326Hexagon is a microservices toolkit written in Kotlin. Its purpose is to ease the building of services (Web applications or APIs) that run inside a cloud platform.
https://github.com/hexagonkt/hexagon
Hexagon is a microservices toolkit written in Kotlin. Its purpose is to ease the building of services (Web applications or APIs) that run inside a cloud platform. - hexagontk/hexagon
- 327Kt. Academy
https://kt.academy/article
Teaching programming, with focus on the best practices.
- 328Minimalist Kotlin dependency injection
https://github.com/traversals/kapsule
Minimalist Kotlin dependency injection. Contribute to gouline/kapsule development by creating an account on GitHub.
- 329The idiomatic way to use atomic operations in Kotlin
https://github.com/Kotlin/kotlinx.atomicfu
The idiomatic way to use atomic operations in Kotlin - Kotlin/kotlinx-atomicfu
- 330Lightweight Multiplatform logging framework for Kotlin. A convenient and performant logging facade.
https://github.com/MicroUtils/kotlin-logging
Lightweight Multiplatform logging framework for Kotlin. A convenient and performant logging facade. - oshai/kotlin-logging
- 331JavaScript syntax highlighter with language auto-detection and zero dependencies.
https://github.com/isagalaev/highlight.js
JavaScript syntax highlighter with language auto-detection and zero dependencies. - highlightjs/highlight.js
- 332KStateMachine is a powerful Kotlin Multiplatform library with clean DSL syntax for creating complex state machines and statecharts driven by Kotlin Coroutines.
https://github.com/nsk90/kstatemachine
KStateMachine is a powerful Kotlin Multiplatform library with clean DSL syntax for creating complex state machines and statecharts driven by Kotlin Coroutines. - KStateMachine/kstatemachine
- 333Advanced Android App Architecture
https://store.raywenderlich.com/products/advanced-android-app-architecture
In Advanced Android App Architectures, you’ll find a diverse and hands-on approach to architecting your apps on Android. Android development can be fun; however, scaling an app can have its fair share of problems. In this book, you’ll learn why a conversation on architecture is the first important step to taking your app to the next level! This book will introduce you to a number of architectures, including Model View Controller, Model View Presenter, Model View Intent, Model-View-ViewModel and VIPER. You’ll learn theory, explore samples that you will refactor and learn the fundamentals of testing.
- 334Kotlin Apprentice
https://store.raywenderlich.com/products/kotlin-apprentice
For complete beginners to Kotlin. No prior programming experience necessary! This is a book for complete beginners to the new, modern Kotlin language. Everything in the book takes place in a clean, modern development environment, which means you can focus on the core features of programming in the Kotlin language, without getting bogged down in the many details of building apps. This is a sister book to the Android Apprentice the Android Apprentice focuses on making apps for Android, while the Kotlin Apprentice focuses on the Kotlin language fundamentals.
- 335Scripting enhancements for Kotlin
https://github.com/holgerbrandl/kscript
Scripting enhancements for Kotlin. Contribute to kscripting/kscript development by creating an account on GitHub.
- 336Server-Side Development with Kotlin
https://www.youtube.com/playlist?list=PLlFc5cFwUnmx-dpq9nkdaVJX0GnrM1Mp1
During our live streams, experts from different companies explain how to use Kotlin for various purposes and answer questions during Q&A sessions.
- 337Android Apprentice
https://store.raywenderlich.com/products/android-apprentice
For Complete Beginners to Android! If you’re completely new to Android or developing in Kotlin, this is the book for you. Android Apprentice takes you all the way from building your first app, to submitting your app for sale. By the end of this book, you’ll be experienced enough to turn your vague ideas into real apps that you can release on the Google Play Store. You’ll build 4 complete apps from scratch — each app is a little more complicated than the previous one. Together, these apps will teach you how to work with the most common controls and APIs used by Android developers around the world. And these aren’t simple apps, either; you’ll build everything from a simple game, to a checklist app, a map-based app, and a podcast manager and player! We’ve also included some bonus sections on handling the Android fragmentation problem, how to keep your app up-to-date, preparing your app for release, testing your app, and publishing it for the world to enjoy! If you’re new to Android programming, you need a guide that: Shows you how to write an app step-by-step With tons of illustrations and screenshots to make everything clear In a fun and easygoing manner! We’ve written this book in Kotlin: the new, modern, first-class language for Android developers. You’ll be at the leading edge of Android developers everywhere as you learn how to develop in the fluid and expressive Kotlin language. The Android Apprentice is your best companion for learning Android development. With all project source code included with the book, and support forums right on our site, it’s simply the best way to start your Android development career.
- 338Data Structures & Algorithms in Kotlin
https://store.raywenderlich.com/products/data-structures-and-algorithms-in-kotlin
Learn Data Structures & Algorithms in Kotlin! Data structures and algorithms are fundamental tools every developer should have. In this book, you’ll learn how to implement key data structures in Kotlin, and how to use them to solve a robust set of algorithms. This book is for intermediate Kotlin or Android developers who already know the basics of the language and want to improve their knowledge. Topics Covered in This Book Introduction to Kotlin: If you’re new to Kotlin, you can learn the main constructs and begin writing code. Complexity: When you study algorithms, you need a way to compare their performance in time and space. Learn about the Big-O notation to help you do this. Elementary Data Structures: Learn how to implement Linked List, Stacks, and Queues in Kotlin. Trees: Learn everything you need about Trees — in particular, Binary Trees, AVL Trees, as well as Binary Search and much more. Sorting Algorithms: Sorting algorithms are critical for any developer. Learn to implement the main sorting algorithms, using the tools provided by Kotlin. Graphs: Have you ever heard of Dijkstra and the calculation of the shortest path between two different points? Learn about Graphs and how to use them to solve the most useful and important algorithms.
- 339Kotlin Course - Tutorial for Beginners
https://youtu.be/F9UC9DY-vIU
Learn the Kotlin programming language in this introduction to Kotlin. Kotlin is a general purpose, open source, statically typed “pragmatic” programming lang...
- 340ReadHub - IntelliJ IDEs Plugin | Marketplace
https://plugins.jetbrains.com/plugin/10539-readhub
Readhub is a News Reader application mainly for Chinese articles Readhub IDE 插件 - 每天高效浏览行业资讯 在 IDE 中快捷查阅科技动态、招聘信息等资讯,有效利用日常工作碎片时间 更多介绍,请访问:GitHub | Issues | Readhub.
- 341Artificial Intelligence planning optimization for Kotlin
https://www.optaplanner.org/compatibility/kotlin.html
Use OptaPlanner (open source) for Artificial Intelligence planning optimization on Kotlin.
- 342RSocket | RSocket
https://rsocket.io
Application protocol providing Reactive Streams semantics
- 343Awesome Kotlin - IntelliJ IDEs Plugin | Marketplace
https://plugins.jetbrains.com/plugin/11357-awesome-kotlin
Awesome Kotlin - A Collection of awesome Kotlin related stuff Show content from Awesome Kotlin in IDE Search / checkout projects from GitHub repo More Info: Plugin...
- 344Planning optimization made easy - Timefold
https://timefold.ai
Experts in PlanningAI for complex scheduling and routing
- 345UNofficial Opengl SDK
https://github.com/kotlin-graphics/uno-sdk
UNofficial Opengl SDK. Contribute to kotlin-graphics/uno-sdk development by creating an account on GitHub.
- 346Kotlin Multiplatform bindings to Skia
https://github.com/JetBrains/skiko
Kotlin Multiplatform bindings to Skia. Contribute to JetBrains/skiko development by creating an account on GitHub.
- 347Three.js port for the JVM (desktop)
https://github.com/markaren/three.kt
Three.js port for the JVM (desktop). Contribute to markaren/three.kt development by creating an account on GitHub.
- 348Aggregated Android news, articles, podcasts and conferences about Android Development
https://github.com/dgngulcan/droid-feed
Aggregated Android news, articles, podcasts and conferences about Android Development - dgngulcan/droid-feed
- 349KickAss Configuration. An annotation-based configuration system for Java and Kotlin
https://github.com/mariomac/kaconf
KickAss Configuration. An annotation-based configuration system for Java and Kotlin - mariomac/kaconf
- 350Reakt is a Kotlin wrapper for facebook's React library
https://github.com/andrewoma/reakt
Reakt is a Kotlin wrapper for facebook's React library - andrewoma/reakt
- 351A simple client for overpass that uses ktor-client and kotlinx.serialization for parsing JSON responses. We are using this at FORMATION to be able to run some simple queries against OpenStreetMap.
https://github.com/formation-res/overpass-kotlin-client
A simple client for overpass that uses ktor-client and kotlinx.serialization for parsing JSON responses. We are using this at FORMATION to be able to run some simple queries against OpenStreetMap. ...
- 352Identify email addresses or domains names that belong to colleges or universities. Help automate the process of approving or rejecting academic discounts.
https://github.com/JetBrains/swot
Identify email addresses or domains names that belong to colleges or universities. Help automate the process of approving or rejecting academic discounts. - JetBrains/swot
- 353Headless Chrome DevTools Protocol Client (RxJava3 + Kotlin)
https://github.com/wendigo/chrome-reactive-kotlin
Headless Chrome DevTools Protocol Client (RxJava3 + Kotlin) - wendigo/chrome-reactive-kotlin
- 354rocketraman/kotlin-web-hello-world
https://github.com/rocketraman/kotlin-web-hello-world
Contribute to rocketraman/kotlin-web-hello-world development by creating an account on GitHub.
- 355forkhandles/bunting4k at trunk · fork-handles/forkhandles
https://github.com/fork-handles/forkhandles/tree/trunk/bunting4k
Foundational libraries for Kotlin. Contribute to fork-handles/forkhandles development by creating an account on GitHub.
- 356Multidimensional array library for Kotlin
https://github.com/Kotlin/multik
Multidimensional array library for Kotlin. Contribute to Kotlin/multik development by creating an account on GitHub.
- 357Type-safe library for work with Telegram Bot API
https://github.com/InsanusMokrassar/ktgbotapi
Type-safe library for work with Telegram Bot API. Contribute to InsanusMokrassar/ktgbotapi development by creating an account on GitHub.
- 358Sigbla is a framework for working with data in tables, using the Kotlin programming language. It supports various data types, reactive programming and events, user input, charts, and more.
https://github.com/sigbla/sigbla-app
Sigbla is a framework for working with data in tables, using the Kotlin programming language. It supports various data types, reactive programming and events, user input, charts, and more. - sigbla...
- 359:closed_umbrella: An easy way to implement modern permission instructions popup.
https://github.com/skydoves/Needs
:closed_umbrella: An easy way to implement modern permission instructions popup. - GitHub - skydoves/Needs: :closed_umbrella: An easy way to implement modern permission instructions popup.
- 360A cross-platform desktop app for hashing data, written in Compose for Desktop
https://github.com/russellbanks/HashHash
A cross-platform desktop app for hashing data, written in Compose for Desktop - russellbanks/HashHash
- 361Kotlin multiplatform logging. High performance, composable and simple to use.
https://github.com/LighthouseGames/KmLogging
Kotlin multiplatform logging. High performance, composable and simple to use. - LighthouseGames/KmLogging
- 362Simple multiplatform logging in Kotlin
https://github.com/InsanusMokrassar/KSLog
Simple multiplatform logging in Kotlin. Contribute to InsanusMokrassar/KSLog development by creating an account on GitHub.
- 363Multiplatform text styling for Kotlin command-line applications
https://github.com/ajalt/mordant
Multiplatform text styling for Kotlin command-line applications - ajalt/mordant
- 364OPENRNDR. A Kotlin/JVM library for creative coding, real-time and interactive graphics
https://github.com/openrndr/openrndr
OPENRNDR. A Kotlin/JVM library for creative coding, real-time and interactive graphics - openrndr/openrndr
- 365Grammar of Graphics for Kotlin
https://github.com/JetBrains/lets-plot-kotlin
Grammar of Graphics for Kotlin. Contribute to JetBrains/lets-plot-kotlin development by creating an account on GitHub.
- 366Multiplatform AWS SDK for Kotlin
https://github.com/awslabs/aws-sdk-kotlin
Multiplatform AWS SDK for Kotlin. Contribute to awslabs/aws-sdk-kotlin development by creating an account on GitHub.
- 367Basic Kotlin Implementation following MVP, and using Third Party library by Natural Analytics Language
https://github.com/cbedoy/DYUM
Basic Kotlin Implementation following MVP, and using Third Party library by Natural Analytics Language - cbedoy/DYUM
- 368A simple Kotlin option parser
https://github.com/jimschubert/kopper
A simple Kotlin option parser. Contribute to jimschubert/kopper development by creating an account on GitHub.
- 369A Kotlin multiplatform coroutine-based SignalR client.
https://github.com/lepicekmichal/SignalRKore
A Kotlin multiplatform coroutine-based SignalR client. - lepicekmichal/SignalRKore
- 370Kotlin plotting library.
https://github.com/Kotlin/kandy
Kotlin plotting library. Contribute to Kotlin/kandy development by creating an account on GitHub.
- 371A Kotlin wrapper for Typesafe Config
https://github.com/config4k/config4k
A Kotlin wrapper for Typesafe Config. Contribute to config4k/config4k development by creating an account on GitHub.
- 372Analytics for Trading with NOA
https://github.com/grinisrit/noa-atra
Analytics for Trading with NOA. Contribute to grinisrit/noa-atra development by creating an account on GitHub.
- 373A scientific computing library for Kotlin. https://kyonifer.github.io/koma
https://github.com/kyonifer/koma
A scientific computing library for Kotlin. https://kyonifer.github.io/koma - kyonifer/koma
- 374Kotlinx coroutines extensions for Java NIO.2
https://github.com/agcom/knio2
Kotlinx coroutines extensions for Java NIO.2. Contribute to agcom/knio2 development by creating an account on GitHub.
- 375High-level Deep Learning Framework written in Kotlin and inspired by Keras
https://github.com/Kotlin/kotlindl
High-level Deep Learning Framework written in Kotlin and inspired by Keras - Kotlin/kotlindl
- 376🗝️ Dotenv is a module that loads environment variables from a .env file
https://github.com/cdimascio/dotenv-kotlin
🗝️ Dotenv is a module that loads environment variables from a .env file - cdimascio/dotenv-kotlin
- 377unsigned support for Kotlin via boxed types and unsigned operators
https://github.com/kotlin-graphics/kotlin-unsigned
unsigned support for Kotlin via boxed types and unsigned operators - kotlin-graphics/kotlin-unsigned
- 378A cross-platform geometry library in Kotlin
https://github.com/cdietze/euklid
A cross-platform geometry library in Kotlin. Contribute to cdietze/euklid development by creating an account on GitHub.
- 379Kotlin/Spring 5 PetClinic application
https://github.com/ssouris/petclinic-spring5-reactive
Kotlin/Spring 5 PetClinic application. Contribute to ssouris/petclinic-spring5-reactive development by creating an account on GitHub.
- 380Multiplatform command line interface parsing for Kotlin
https://github.com/ajalt/clikt
Multiplatform command line interface parsing for Kotlin - ajalt/clikt
- 381Structured data processing in Kotlin
https://github.com/Kotlin/dataframe
Structured data processing in Kotlin. Contribute to Kotlin/dataframe development by creating an account on GitHub.
- 382JVM Open Asset Import Library (Assimp)
https://github.com/kotlin-graphics/assimp
JVM Open Asset Import Library (Assimp). Contribute to kotlin-graphics/assimp development by creating an account on GitHub.
- 383Barber 💈 A type safe Kotlin JVM library for building up localized, fillable, themed documents using Mustache templating
https://github.com/cashapp/barber
Barber 💈 A type safe Kotlin JVM library for building up localized, fillable, themed documents using Mustache templating - cashapp/barber
- 384Neumorphism UI on Android with Jetpack Compose.
https://github.com/CuriousNikhil/neumorphic-compose
Neumorphism UI on Android with Jetpack Compose. Contribute to CuriousNikhil/neumorphic-compose development by creating an account on GitHub.
- 385Quickly compile Kotlin classes (.kt) and run Kotlin scripts (.kts)
https://github.com/hazae41/Kotlin-Compiler-GUI
Quickly compile Kotlin classes (.kt) and run Kotlin scripts (.kts) - hazae41/kotlin-compiler-gui
- 386A collection of common interactive command line user interfaces written in Kotlin
https://github.com/kotlin-inquirer/kotlin-inquirer
A collection of common interactive command line user interfaces written in Kotlin - kotlin-inquirer/kotlin-inquirer
- 387Rankquest Studio is a web based tool that you can use to benchmark search relevance metrics for your search APIs.
https://github.com/jillesvangurp/rankquest-studio
Rankquest Studio is a web based tool that you can use to benchmark search relevance metrics for your search APIs. - jillesvangurp/rankquest-studio
- 388Spring Boot Kotlin project with a REST Webservice and Spring Data
https://github.com/sdeleuze/spring-boot-kotlin-demo
Spring Boot Kotlin project with a REST Webservice and Spring Data - sdeleuze/spring-boot-kotlin-demo
- 389Open source Crypto Currency Tracker Android App made fully in Kotlin
https://github.com/pranayairan/CoinBit
Open source Crypto Currency Tracker Android App made fully in Kotlin - GitHub - pranayairan/CoinBit: Open source Crypto Currency Tracker Android App made fully in Kotlin
- 390A type-safe cascading configuration library for Kotlin/Java/Android, supporting most configuration formats
https://github.com/uchuhimo/konf
A type-safe cascading configuration library for Kotlin/Java/Android, supporting most configuration formats - uchuhimo/konf
- 391Spotify Web API wrapper for Kotlin, Java, JS, and Native - Targets JVM, Android, JS (browser), Native (Desktop), and Apple tvOS/iOS. Includes a Spotify Web Playback SDK wrapper for Kotlin/JS, and a spotify-auth wrapper for Kotlin/Android.
https://github.com/adamint/spotify-web-api-kotlin
Spotify Web API wrapper for Kotlin, Java, JS, and Native - Targets JVM, Android, JS (browser), Native (Desktop), and Apple tvOS/iOS. Includes a Spotify Web Playback SDK wrapper for Kotlin/JS, and a...
- 392kotlin-graphics/ovr
https://github.com/kotlin-graphics/ovr
Contribute to kotlin-graphics/ovr development by creating an account on GitHub.
- 393An example of a Kotlin Ratpack app built with Gradle.
https://github.com/ratpack/example-ratpack-gradle-kotlin-app
An example of a Kotlin Ratpack app built with Gradle. - ratpack/example-ratpack-gradle-kotlin-app
- 394A kotlin multiplatform wrapper for libsodium, using directly built libsodium for jvm and native, and libsodium.js for js targets.
https://github.com/ionspin/kotlin-multiplatform-libsodium
A kotlin multiplatform wrapper for libsodium, using directly built libsodium for jvm and native, and libsodium.js for js targets. - ionspin/kotlin-multiplatform-libsodium
- 395async/await for Android built upon coroutines introduced in Kotlin 1.1
https://github.com/metalabdesign/AsyncAwait
async/await for Android built upon coroutines introduced in Kotlin 1.1 - metalabdesign/AsyncAwait
- 396Computer Vision on Android with Kotlin and Tensorflow Lite
https://github.com/eddywm/KTFLITE
Computer Vision on Android with Kotlin and Tensorflow Lite - eddywm/KTFLITE
- 397Easy app for managing your files without ads, respecting your privacy & security
https://github.com/SimpleMobileTools/Simple-File-Manager
Easy app for managing your files without ads, respecting your privacy & security - SimpleMobileTools/Simple-File-Manager
- 398🏀 An Android app for dribbble.com
https://github.com/TonnyL/Mango
🏀 An Android app for dribbble.com. Contribute to TonnyL/Mango development by creating an account on GitHub.
- 399Seed project for facebook messenger bots. Vertx, Kotlin.
https://github.com/ivanpopelyshev/vertx-facebook-messenger
Seed project for facebook messenger bots. Vertx, Kotlin. - ivanpopelyshev/vertx-facebook-messenger
- 400A Kotlin multi-platform library for graph data structures
https://github.com/lamba92/KGraph
A Kotlin multi-platform library for graph data structures - lamba92/KGraph
- 401Companion App for the book
https://github.com/antoniolg/Kotlin-for-Android-Developers
Companion App for the book. Contribute to antoniolg/Kotlin-for-Android-Developers development by creating an account on GitHub.
- 402101 examples for Kotlin Programming language.
https://github.com/dodyg/Kotlin101
101 examples for Kotlin Programming language. Contribute to dodyg/Kotlin101 development by creating an account on GitHub.
- 403A Kotlin Multiplatform Mobile App
https://github.com/itmaginationdemos/KMM-Sample-App
A Kotlin Multiplatform Mobile App. Contribute to itmaginationdemos/KMM-Sample-App development by creating an account on GitHub.
- 404High-performance, low-cost microservice governance platform. Service Discovery and Configuration Service | 高性能、低成本微服务治理平台
https://github.com/Ahoo-Wang/CoSky
High-performance, low-cost microservice governance platform. Service Discovery and Configuration Service | 高性能、低成本微服务治理平台 - Ahoo-Wang/CoSky
- 405Kotlin DSL для разработки Telegram ботов
https://github.com/ruslanys/telegraff
Kotlin DSL для разработки Telegram ботов. Contribute to ruslanys/telegraff development by creating an account on GitHub.
- 406Demo of an asynchronous app for long-response-time web services.
https://github.com/mariomac/codebuilder
Demo of an asynchronous app for long-response-time web services. - mariomac/codebuilder
- 407Simple config properties API for Kotlin
https://github.com/npryce/konfig
Simple config properties API for Kotlin. Contribute to npryce/konfig development by creating an account on GitHub.
- 408Kodein adapters for several FaaS providers
https://github.com/viniciusccarvalho/kodein-cloud-functions
Kodein adapters for several FaaS providers. Contribute to viniciusccarvalho/kodein-cloud-functions development by creating an account on GitHub.
- 409OpenVR SDK
https://github.com/kotlin-graphics/openvr
OpenVR SDK. Contribute to kotlin-graphics/openvr development by creating an account on GitHub.
- 410:cyclone: A Pokedex app using ViewModel, ViewBinding, LiveData, Room and Navigation
https://github.com/mrcsxsiq/Kotlin-Pokedex
:cyclone: A Pokedex app using ViewModel, ViewBinding, LiveData, Room and Navigation - mrcsxsiq/Kotlin-Pokedex
- 411Example of Android project in Kotlin using MVP pattern and volley library
https://github.com/emedinaa/kotlin-mvp-volley
Example of Android project in Kotlin using MVP pattern and volley library - emedinaa/kotlin-mvp-volley
- 412Android News Reader app. Kotlin Coroutines, Retrofit and Realm
https://github.com/deva666/NewsReader
Android News Reader app. Kotlin Coroutines, Retrofit and Realm - deva666/NewsReader
- 413Swingin’ filler text for your jet-age web page.
https://github.com/robfletcher/midcentury-ipsum
Swingin’ filler text for your jet-age web page. Contribute to robfletcher/midcentury-ipsum development by creating an account on GitHub.
- 414Kotlin workshop
https://github.com/Kotlin/kotlin-koans
Kotlin workshop. Contribute to Kotlin/kotlin-koans development by creating an account on GitHub.
- 415A canvas you can draw on with different colors.
https://github.com/SimpleMobileTools/Simple-Draw
A canvas you can draw on with different colors. Contribute to SimpleMobileTools/Simple-Draw development by creating an account on GitHub.
- 416Intelij IDEA plugin for displaying a code mini-map similar to the one found in Sublime
https://github.com/Vektah/CodeGlance
Intelij IDEA plugin for displaying a code mini-map similar to the one found in Sublime - vektah/CodeGlance
- 417📐 Visualize the dimensions of your composables on a blueprint!
https://github.com/popovanton0/Blueprint
📐 Visualize the dimensions of your composables on a blueprint! - popovanton0/Blueprint
- 418A lightweight particle system for Jetpack Compose - Quarks
https://github.com/CuriousNikhil/compose-particle-system
A lightweight particle system for Jetpack Compose - Quarks - CuriousNikhil/compose-particle-system
- 419Type-safe Kotlin configuration by delegates
https://github.com/aPureBase/arkenv
Type-safe Kotlin configuration by delegates. Contribute to aPureBase/arkenv development by creating an account on GitHub.
- 420Konclik: Kotlin/Native Command Line Interface Kit
https://github.com/dbaelz/Konclik
Konclik: Kotlin/Native Command Line Interface Kit. Contribute to dbaelz/Konclik development by creating an account on GitHub.
- 421kotlin extensions to the java8 time library
https://github.com/hankdavidson/ktime
kotlin extensions to the java8 time library. Contribute to hankdavidson/ktime development by creating an account on GitHub.
- 422Zero-overhead 2D rendering library for JOGL using Kotlin
https://github.com/Jonatino/JOGL2D
Zero-overhead 2D rendering library for JOGL using Kotlin - jonatino/JOGL2D
- 423JVM Bullet Physics SDK: real-time collision detection and multi-physics simulation for VR, games, visual effects, robotics, machine learning etc.
https://github.com/kotlin-graphics/bullet
JVM Bullet Physics SDK: real-time collision detection and multi-physics simulation for VR, games, visual effects, robotics, machine learning etc. - GitHub - kotlin-graphics/bullet: JVM Bullet Phys...
- 424Komputation is a neural network framework for the Java Virtual Machine written in Kotlin and CUDA C.
https://github.com/sekwiatkowski/Komputation
Komputation is a neural network framework for the Java Virtual Machine written in Kotlin and CUDA C. - sekwiatkowski/komputation
- 425Kotlin tooling implementation of ProjectFluent
https://github.com/projectfluent/fluent-kotlin
Kotlin tooling implementation of ProjectFluent. Contribute to projectfluent/fluent-kotlin development by creating an account on GitHub.
- 426jvm gli
https://github.com/kotlin-graphics/gli
jvm gli. Contribute to kotlin-graphics/gli development by creating an account on GitHub.
- 427A smart colored time selector. Users can select just free time with a handy colorful range selector.
https://github.com/ehsunshine/colored-time-selector
A smart colored time selector. Users can select just free time with a handy colorful range selector. - ehsunshine/colored-time-selector
- 428A curated collection of splendid gradients made in Kotlin
https://github.com/bakhtiyork/gradients
A curated collection of splendid gradients made in Kotlin - bakhtiyork/gradients
- 429Compose multiple Adapter for RecyclerView in Android
https://github.com/Jintin/MixAdapter
Compose multiple Adapter for RecyclerView in Android - Jintin/MixAdapter
- 430A lightweight event bus written in Kotlin and RxJava
https://github.com/yundom/RxVan
A lightweight event bus written in Kotlin and RxJava - yundom/RxVan
- 431Bot for my friends
https://github.com/AngryJKirk/familybot
Bot for my friends. Contribute to AngryJKirk/familybot development by creating an account on GitHub.
- 432IntelliJ plugin to limit your work-in-progress
https://github.com/dkandalov/limited-wip
IntelliJ plugin to limit your work-in-progress. Contribute to dkandalov/limited-wip development by creating an account on GitHub.
- 433Pomodoro timer for IntelliJ 🍅⏲
https://github.com/dkandalov/pomodoro-tm
Pomodoro timer for IntelliJ 🍅⏲. Contribute to dkandalov/pomodoro-tm development by creating an account on GitHub.
- 434Analytics library preferably sharpened for Google Analytics
https://github.com/programmerr47/ganalytics
Analytics library preferably sharpened for Google Analytics - programmerr47/ganalytics
- 435Basic samples about ViewModel component
https://github.com/emedinaa/kotlin-viewmodel
Basic samples about ViewModel component. Contribute to emedinaa/kotlin-viewmodel development by creating an account on GitHub.
- 436TodoMVC implementation in React and Kotlin
https://github.com/mkraynov/todomvc-react-kotlin
TodoMVC implementation in React and Kotlin. Contribute to mkraynov/todomvc-react-kotlin development by creating an account on GitHub.
- 437A Kotlin DSL for regular expressions
https://github.com/h0tk3y/regex-dsl
A Kotlin DSL for regular expressions. Contribute to h0tk3y/regex-dsl development by creating an account on GitHub.
- 438Multi platform kotlin client for Elasticsearch & Opensearch with easily extendable Kotlin DSLs for queries, mappings, bulk, and more.
https://github.com/jillesvangurp/kt-search
Multi platform kotlin client for Elasticsearch & Opensearch with easily extendable Kotlin DSLs for queries, mappings, bulk, and more. - jillesvangurp/kt-search
- 439arbitrage finder
https://github.com/FirstArtaxer/karbitrage
arbitrage finder. Contribute to FirstArtaxer/karbitrage development by creating an account on GitHub.
- 440Nano-library which provides the ability to define typesafe (!) configuration templates for applications.
https://github.com/daviddenton/configur8
Nano-library which provides the ability to define typesafe (!) configuration templates for applications. - daviddenton/configur8
- 441A Multiplatform Kotlin SVG image DSL.
https://github.com/nwillc/ksvg
A Multiplatform Kotlin SVG image DSL. Contribute to nwillc/ksvg development by creating an account on GitHub.
- 442MiXiT website
https://github.com/mixitconf/mixit
MiXiT website. Contribute to mixitconf/mixit development by creating an account on GitHub.
- 443Kotlin multi platdform localization for js and jvm based on project fluent
https://github.com/formation-res/fluent-kotlin
Kotlin multi platdform localization for js and jvm based on project fluent - formation-res/fluent-kotlin
- 444View "injection" library for Android.
https://github.com/JakeWharton/kotterknife
View "injection" library for Android. Contribute to JakeWharton/kotterknife development by creating an account on GitHub.
- 445Starter for Spring Boot 2.0 Kotlin applications with configurations for Gradle, Mongo, JUnit 5 tests, logging, CircleCI and Docker compose
https://github.com/yyunikov/spring-boot-2-kotlin-starter
Starter for Spring Boot 2.0 Kotlin applications with configurations for Gradle, Mongo, JUnit 5 tests, logging, CircleCI and Docker compose - yyunikov/spring-boot-2-kotlin-starter
- 446jvm glm
https://github.com/kotlin-graphics/glm
jvm glm. Contribute to kotlin-graphics/glm development by creating an account on GitHub.
- 447A playground to gain a wider and deeper knowledge of the libraries in the Kotlin ecosystem. Also the official sample for gradle refreshVersions.
https://github.com/LouisCAD/kotlin-libraries-playground
A playground to gain a wider and deeper knowledge of the libraries in the Kotlin ecosystem. Also the official sample for gradle refreshVersions. - LouisCAD/kotlin-libraries-playground
- 448Plugin for IntelliJ IDEs to track and record user activity
https://github.com/dkandalov/activity-tracker
Plugin for IntelliJ IDEs to track and record user activity - dkandalov/activity-tracker
- 449A plugin that adds errors / hints / quickfix related to checked exceptions for kotlin.
https://github.com/csense-oss/idea-kotlin-checked-exceptions
A plugin that adds errors / hints / quickfix related to checked exceptions for kotlin. - GitHub - csense-oss/idea-kotlin-checked-exceptions: A plugin that adds errors / hints / quickfix related to...
- 450:musical_score: A materially designed music player
https://github.com/ice1000/Dekoder
:musical_score: A materially designed music player - ice1000/Dekoder
- 451Android preferences made easy
https://github.com/calintat/alps
Android preferences made easy. Contribute to calintat/alps development by creating an account on GitHub.
- 452Example of Android project showing integration with Kotlin and Dagger 2
https://github.com/damianpetla/kotlin-dagger-example
Example of Android project showing integration with Kotlin and Dagger 2 - damianpetla/kotlin-dagger-example
- 453Kabu generates code for complex Kotlin DSLs in less than 1 minute
https://github.com/bipokot/Kabu
Kabu generates code for complex Kotlin DSLs in less than 1 minute - bipokot/Kabu
- 454Rust plugin for the IntelliJ Platform
https://github.com/intellij-rust/intellij-rust
Rust plugin for the IntelliJ Platform. Contribute to intellij-rust/intellij-rust development by creating an account on GitHub.
- 455Write your GLSL shaders in Kotlin.
https://github.com/dananas/kotlin-glsl
Write your GLSL shaders in Kotlin. Contribute to dananas/kotlin-glsl development by creating an account on GitHub.
- 456A JVM implementation of the Pair Adjacent Violators algorithm for isotonic regression
https://github.com/sanity/pairAdjacentViolators
A JVM implementation of the Pair Adjacent Violators algorithm for isotonic regression - sanity/pairAdjacentViolators
- 457Simple Kotlin and Java configuration library with recursive placeholders resolution and zero magic!
https://github.com/ufoscout/properlty
Simple Kotlin and Java configuration library with recursive placeholders resolution and zero magic! - ufoscout/properlty
- 458Android MVVM framework write in kotlin, develop Android has never been so fun.
https://github.com/BennyWang/KBinding
Android MVVM framework write in kotlin, develop Android has never been so fun. - BennyWang/KBinding
- 459Environment analysis tool
https://github.com/Kotlin/kdoctor
Environment analysis tool. Contribute to Kotlin/kdoctor development by creating an account on GitHub.
- 460This lib implements the most common CoroutineScopes used in Android apps.
https://github.com/adrielcafe/AndroidCoroutineScopes
This lib implements the most common CoroutineScopes used in Android apps. - adrielcafe/AndroidCoroutineScopes
- 461A collection of Kotlin Multiplatform cryptographic hashing functions.
https://github.com/appmattus/crypto
A collection of Kotlin Multiplatform cryptographic hashing functions. - appmattus/crypto
- 462RxJava wrapper for NeuroSky MindWave headsets
https://github.com/hpost/rx-brainwaves
RxJava wrapper for NeuroSky MindWave headsets. Contribute to hpost/rx-brainwaves development by creating an account on GitHub.
- 463Android Kotlin "Virtual DOM"
https://github.com/robinchew/mvil
Android Kotlin "Virtual DOM". Contribute to robinchew/mvil development by creating an account on GitHub.
- 464SharedPreference usage made fun in Kotlin
https://github.com/MarcinMoskala/PreferenceHolder
SharedPreference usage made fun in Kotlin. Contribute to MarcinMoskala/PreferenceHolder development by creating an account on GitHub.
- 465Android utilities for easier and faster Kotlin programming.
https://github.com/costular/kotlin-android-utils
Android utilities for easier and faster Kotlin programming. - costular/kotlin-android-utils
- 466:crystal_ball: Single file Kotlin/Java IDE features in lightweight.
https://github.com/ice1000/dev-kt
:crystal_ball: Single file Kotlin/Java IDE features in lightweight. - ice1000/dev-kt
- 467📦📦Video downloader for Android - Download videos from Youtube, Facebook, Twitter, Instagram, Dailymotion, Vimeo and more than 1000 other sites
https://github.com/cuongpm/youtube-dl-android
📦📦Video downloader for Android - Download videos from Youtube, Facebook, Twitter, Instagram, Dailymotion, Vimeo and more than 1000 other sites - cuongpm/youtube-dl-android
- 468Music synthesizer, written for fun and to learn stuff
https://github.com/krzema12/fsynth
Music synthesizer, written for fun and to learn stuff - fsynthlib/fsynth
- 469The real life Command/Ctrl + F - Android App that uses the Mobile Vision API to allow you to search for any occurrence of a text in a digital document
https://github.com/WilderPereira/ftwfy
The real life Command/Ctrl + F - Android App that uses the Mobile Vision API to allow you to search for any occurrence of a text in a digital document - WilderPereira/ftwfy
- 470A premium app for managing and editing your photos, videos, GIFs without ads
https://github.com/SimpleMobileTools/Simple-Gallery
A premium app for managing and editing your photos, videos, GIFs without ads - SimpleMobileTools/Simple-Gallery
- 471A fork of our clean architecture boilerplate, this time using the Android Architecture Components
https://github.com/bufferapp/clean-architecture-components-boilerplate
A fork of our clean architecture boilerplate, this time using the Android Architecture Components - bufferapp/clean-architecture-components-boilerplate
- 472Set of extensions for Kotlin that provides Discrete math functionalities
https://github.com/MarcinMoskala/KotlinDiscreteMathToolkit
Set of extensions for Kotlin that provides Discrete math functionalities - MarcinMoskala/KotlinDiscreteMathToolkit
- 473Companion to Kotlin standard library
https://github.com/LukasForst/katlib
Companion to Kotlin standard library. Contribute to LukasForst/katlib development by creating an account on GitHub.
- 474Multiplatform wavy slider/progress bar similar to the one in Android 13
https://github.com/mahozad/wavy-slider
Multiplatform wavy slider/progress bar similar to the one in Android 13 - mahozad/wavy-slider
- 475Flexible and easy to use config library written in kotlin
https://github.com/jdiazcano/cfg4k
Flexible and easy to use config library written in kotlin - jdiazcano/cfg4k
- 476Celebrate more with this lightweight confetti particle system 🎊
https://github.com/DanielMartinus/Konfetti
Celebrate more with this lightweight confetti particle system 🎊 - DanielMartinus/Konfetti
- 477Dead simple EventBus for Android made with Kotlin and RxJava 2
https://github.com/adrielcafe/KBus
Dead simple EventBus for Android made with Kotlin and RxJava 2 - adrielcafe/KBus
- 478A collection of useful Kotlin Extension
https://github.com/vanshg/KrazyKotlin
A collection of useful Kotlin Extension. Contribute to vanshg/KrazyKotlin development by creating an account on GitHub.
- 479Provides the Kotlin plugin via the Gradle plugin portal, automatically depends on the standard library, and allows Kotlin library versions to be omitted
https://github.com/nebula-plugins/nebula-kotlin-plugin
Provides the Kotlin plugin via the Gradle plugin portal, automatically depends on the standard library, and allows Kotlin library versions to be omitted - nebula-plugins/nebula-kotlin-plugin
- 480Simple android library to present an animated ferris wheel
https://github.com/iglaweb/Ferris-Wheel
Simple android library to present an animated ferris wheel - iglaweb/Ferris-Wheel
- 481Android Library to make SharedPreferences usage easier.
https://github.com/MarcinMoskala/KotlinPreferences
Android Library to make SharedPreferences usage easier. - MarcinMoskala/KotlinPreferences
- 482Kotlin Examples Problems
https://github.com/vicboma1/Kotlin-Examples-Problems
Kotlin Examples Problems. Contribute to vicboma1/Kotlin-Examples-Problems development by creating an account on GitHub.
- 483Integration Testing Kotlin Multiplatform Kata for Kotlin Developers. The main goal is to practice integration testing using Ktor and Ktor Client Mock
https://github.com/xurxodev/integration-testing-kotlin-multiplatform-kata
Integration Testing Kotlin Multiplatform Kata for Kotlin Developers. The main goal is to practice integration testing using Ktor and Ktor Client Mock - xurxodev/integration-testing-kotlin-multiplat...
- 484📒 NotyKT is a complete 💎Kotlin-stack (Backend + Android) 📱 application built to demonstrate the use of Modern development tools with best practices implementation🦸.
https://github.com/PatilShreyas/NotyKT
📒 NotyKT is a complete 💎Kotlin-stack (Backend + Android) 📱 application built to demonstrate the use of Modern development tools with best practices implementation🦸. - PatilShreyas/NotyKT
- 485Currency Exchange App that displays real-time currency rates, shows a chart for any currency pair in the world to see their currency history and provides a currency converter to convert over 180 currencies implemented in MVVM Architecture using Koltin.
https://github.com/Marwa-Eltayeb/CurrencyExchange
Currency Exchange App that displays real-time currency rates, shows a chart for any currency pair in the world to see their currency history and provides a currency converter to convert over 180 cu...
- 486A simple calendar with events, tasks, customizable colors, widgets and no ads.
https://github.com/SimpleMobileTools/Simple-Calendar
A simple calendar with events, tasks, customizable colors, widgets and no ads. - SimpleMobileTools/Simple-Calendar
- 487Nice and simple DSL for Espresso in Kotlin
https://github.com/KakaoCup/Kakao
Nice and simple DSL for Espresso in Kotlin. Contribute to KakaoCup/Kakao development by creating an account on GitHub.
- 488Don't write a RecyclerView adapter again. Not even a ViewHolder!
https://github.com/nitrico/LastAdapter
Don't write a RecyclerView adapter again. Not even a ViewHolder! - nitrico/LastAdapter
- 489Android sample with kotlin.
https://github.com/wangjiegulu/KotlinAndroidSample
Android sample with kotlin. Contribute to wangjiegulu/KotlinAndroidSample development by creating an account on GitHub.
- 490Examples with Kotlin/Wasm
https://github.com/Kotlin/kotlin-wasm-examples
Examples with Kotlin/Wasm. Contribute to Kotlin/kotlin-wasm-examples development by creating an account on GitHub.
- 491Demo for July 2022 Kotlin Virtual User Group
https://github.com/formation-res/kt-fullstack-demo
Demo for July 2022 Kotlin Virtual User Group. Contribute to formation-res/kt-fullstack-demo development by creating an account on GitHub.
- 492Easy lightweight SharedPreferences library for Android in Kotlin using delegated properties
https://github.com/mathcamp/fiberglass
Easy lightweight SharedPreferences library for Android in Kotlin using delegated properties - mathcamp/fiberglass
- 493Stateful is a Kotlin library which makes Android application development faster and easier.
https://github.com/PicsArt/stateful
Stateful is a Kotlin library which makes Android application development faster and easier. - PicsArt/stateful
- 494A simple android Twitter client written in Kotlin
https://github.com/ziggy42/Blum-kotlin
A simple android Twitter client written in Kotlin. Contribute to ziggy42/Blum development by creating an account on GitHub.
- 495JAlgoArena programming contest platform
https://github.com/spolnik/JAlgoArena
JAlgoArena programming contest platform. Contribute to jalgoarena/JAlgoArena development by creating an account on GitHub.
- 496A showcase music app for Android entirely written using Kotlin language
https://github.com/antoniolg/Bandhook-Kotlin
A showcase music app for Android entirely written using Kotlin language - antoniolg/Bandhook-Kotlin
- 497Kaffeine is a Kotlin-flavored Android library for accelerating development.
https://github.com/ragunathjawahar/kaffeine
Kaffeine is a Kotlin-flavored Android library for accelerating development. - ragunathjawahar/kaffeine
- 498Glimpse is now further developed as a Kotlin Multiplatform project under glimpse-graphics/glimpse
https://github.com/GlimpseFramework/glimpse-framework-android
Glimpse is now further developed as a Kotlin Multiplatform project under glimpse-graphics/glimpse - glimpse-graphics/glimpse-framework-android
- 499Kotlin webpack loader
https://github.com/huston007/kotlin-loader
Kotlin webpack loader. Contribute to andrey-skl/kotlin-loader development by creating an account on GitHub.
- 500MVVM(Model View ViewModel) sample in Kotlin using the components ViewModel, LiveData and Retrofit library
https://github.com/emedinaa/kotlin-mvvm
MVVM(Model View ViewModel) sample in Kotlin using the components ViewModel, LiveData and Retrofit library - emedinaa/kotlin-mvvm
- 501C++ examples for the Vulkan graphics API
https://github.com/SaschaWillems/Vulkan
C++ examples for the Vulkan graphics API. Contribute to SaschaWillems/Vulkan development by creating an account on GitHub.
- 502Kotlin client for JetBrains Space HTTP API
https://github.com/JetBrains/space-kotlin-sdk
Kotlin client for JetBrains Space HTTP API. Contribute to JetBrains/space-kotlin-sdk development by creating an account on GitHub.
- 503Kotlin library for Android
https://github.com/pawegio/KAndroid
Kotlin library for Android. Contribute to pawegio/KAndroid development by creating an account on GitHub.
- 504Functional Constructs for Databinding + Kotlin + RxJava
https://github.com/rakshakhegde/ObservableFlow
Functional Constructs for Databinding + Kotlin + RxJava - rakshakhegde/ObservableFlow
- 505A lightweight cache library written in Kotlin
https://github.com/yundom/kache
A lightweight cache library written in Kotlin. Contribute to yundom/kache development by creating an account on GitHub.
- 506🛠️ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files.
https://github.com/duanhong169/DrawableToolbox
🛠️ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. - duanhong169/DrawableToolbox
- 507:pencil2: Method call logging for Kotlin Multiplatform
https://github.com/Foso/Cabret-Log
:pencil2: Method call logging for Kotlin Multiplatform - Foso/Cabret-Log
- 508Ostara is a cross-platform desktop app for managing and monitoring Spring Boot applications using the Actuator API, providing comprehensive insights and effortless control.
https://github.com/krud-dev/ostara
Ostara is a cross-platform desktop app for managing and monitoring Spring Boot applications using the Actuator API, providing comprehensive insights and effortless control. - krud-dev/ostara
- 509:octocat: A demo project based on MVVM architecture and material design & animations.
https://github.com/skydoves/githubfollows
:octocat: A demo project based on MVVM architecture and material design & animations. - skydoves/GithubFollows
- 510Android Library that provide simpler way to achieve modularity
https://github.com/SnowdreamFramework/ToyBricks
Android Library that provide simpler way to achieve modularity - SnowdreamFramework/ToyBricks
- 511DSL for constructing the drawables in Kotlin instead of in XML
https://github.com/infotech-group/android-drawable-dsl
DSL for constructing the drawables in Kotlin instead of in XML - infotech-group/android-drawable-dsl
- 512A simple Kotlin wrapper around Anvil.
https://github.com/andre-artus/AnvilKotlin
A simple Kotlin wrapper around Anvil. Contribute to andre-artus/AnvilKotlin development by creating an account on GitHub.
- 513Screen orientation detector for android
https://github.com/TouK/bubble
Screen orientation detector for android. Contribute to TouK/bubble development by creating an account on GitHub.
- 514Android RecyclerView
https://github.com/emedinaa/android-recyclerview
Android RecyclerView. Contribute to emedinaa/android-recyclerview development by creating an account on GitHub.
- 515A collaborative project to create a Free and Open Source Buddha Quotes app for Android
https://github.com/BanDev/Buddha-Quotes
A collaborative project to create a Free and Open Source Buddha Quotes app for Android - BanDev/BuddhaQuotes
- 516🔥🖼 Display images stored in Cloud Storage for Firebase using Coil
https://github.com/rosariopfernandes/firecoil
🔥🖼 Display images stored in Cloud Storage for Firebase using Coil - thatfiredev/firecoil
- 517AwesomeKotlin IntelliJ Plugin
https://github.com/roger-yh99/AwesomeKotlinPlugin
AwesomeKotlin IntelliJ Plugin. Contribute to yaohui-wyh/AwesomeKotlinPlugin development by creating an account on GitHub.
- 518An android boilerplate project using clean architecture
https://github.com/bufferapp/android-clean-architecture-boilerplate
An android boilerplate project using clean architecture - bufferapp/android-clean-architecture-boilerplate
- 519Android app that shows what happened today in the history.
https://github.com/MakinGiants/todayhistory
Android app that shows what happened today in the history. - MakinGiants/todayhistory
- 520Screenshot Kata for Android Developers with Kotlin. The main goal is to practice UI Screenshot Testing.
https://github.com/Karumi/KataScreenshotKotlin
Screenshot Kata for Android Developers with Kotlin. The main goal is to practice UI Screenshot Testing. - Karumi/KataScreenshotKotlin
- 521TwidereProject/Twidere-Android
https://github.com/TwidereProject/Twidere-Android
Contribute to TwidereProject/Twidere-Android development by creating an account on GitHub.
- 522PSP Emulator written in Kotlin for JVM, JS and Native. Can work as PWA.
https://github.com/kpspemu/kpspemu
PSP Emulator written in Kotlin for JVM, JS and Native. Can work as PWA. - kpspemu/kpspemu
- 523Приложение для выгрузки аудио библиотеки из ВК
https://github.com/ruslanys/vkmusic
Приложение для выгрузки аудио библиотеки из ВК. Contribute to ruslanys/vkmusic development by creating an account on GitHub.
- 524Kode-First Konfiguration for Kotlin
https://github.com/davidohana/kofiko-kotlin
Kode-First Konfiguration for Kotlin. Contribute to davidohana/kofiko-kotlin development by creating an account on GitHub.
- 525A Kotlin DSL wrapper around the mikepenz/MaterialDrawer library.
https://github.com/zsmb13/MaterialDrawerKt
A Kotlin DSL wrapper around the mikepenz/MaterialDrawer library. - zsmb13/MaterialDrawerKt
- 526An Android Library that provides social login for 15 platforms within by RxJava2, Kotlin and Firebase Authentication.
https://github.com/WindSekirun/RxSocialLogin
An Android Library that provides social login for 15 platforms within by RxJava2, Kotlin and Firebase Authentication. - GitHub - WindSekirun/RxSocialLogin: An Android Library that provides social ...
- 527Kotlin/Native infrastructure
https://github.com/JetBrains/kotlin-native
Kotlin/Native infrastructure. Contribute to JetBrains/kotlin-native development by creating an account on GitHub.
- 528A collection of useful extension methods for Android
https://github.com/nsk-mironov/kotlin-jetpack
A collection of useful extension methods for Android - nsk-mironov/kotlin-jetpack
- 529Kotlin-CLI - command line interface options parser for Kotlin
https://github.com/leprosus/kotlin-cli
Kotlin-CLI - command line interface options parser for Kotlin - leprosus/kotlin-cli
- 530Clay is an Android library project that provides image trimming which is originally an UI component of LINE Creators Studio
https://github.com/line/clay
Clay is an Android library project that provides image trimming which is originally an UI component of LINE Creators Studio - line/clay
- 531Stepper Touch for Android based on MaterialUp submission
https://github.com/DanielMartinus/Stepper-Touch
Stepper Touch for Android based on MaterialUp submission - DanielMartinus/Stepper-Touch
- 532A simple textfield for adding quick notes without ads.
https://github.com/SimpleMobileTools/Simple-Notes
A simple textfield for adding quick notes without ads. - SimpleMobileTools/Simple-Notes
- 533Super Heroes Kata for Android Developers in Kotlin. The main goal is to practice UI Testing.
https://github.com/Karumi/KataSuperHeroesKotlin
Super Heroes Kata for Android Developers in Kotlin. The main goal is to practice UI Testing. - Karumi/KataSuperHeroesKotlin
- 534OTPComposable is an Android library dedicated to making OTP (One Time Password) components a breeze to implement in Android apps.
https://github.com/itmaginationdemos/OTPComposable
OTPComposable is an Android library dedicated to making OTP (One Time Password) components a breeze to implement in Android apps. - itmaginationdemos/OTPComposable
- 535LinkHub is a simple and effective link management application that can help you to easily manage your app with no ads!
https://github.com/AmrDeveloper/Linkhub
LinkHub is a simple and effective link management application that can help you to easily manage your app with no ads! - GitHub - AmrDeveloper/LinkHub: LinkHub is a simple and effective link manag...
- 536Android AsyncTask wrapper library, written in Kotlin
https://github.com/inaka/KillerTask
Android AsyncTask wrapper library, written in Kotlin - inaka/KillerTask
- 537Kotlin Full-stack Application Example
https://github.com/Kotlin/kotlin-fullstack-sample
Kotlin Full-stack Application Example. Contribute to Kotlin/kotlin-fullstack-sample development by creating an account on GitHub.
- 538Quick photo and video camera with a flash, customizable aspect ratio.
https://github.com/SimpleMobileTools/Simple-Camera
Quick photo and video camera with a flash, customizable aspect ratio. - SimpleMobileTools/Simple-Camera
- 539Easy to use, high level framework in Kotlin for front-end web-development
https://github.com/Kabbura/Kunafa
Easy to use, high level framework in Kotlin for front-end web-development - Narbase/Kunafa
- 540Readhub IntelliJ Plugin
https://github.com/roger-yh99/Readhub
Readhub IntelliJ Plugin. Contribute to yaohui-wyh/Readhub development by creating an account on GitHub.
- 541Tyzenhaus is a shared expenses tracking bot.
https://github.com/madhead/tyzenhaus
Tyzenhaus is a shared expenses tracking bot. Contribute to madhead/tyzenhaus development by creating an account on GitHub.
- 542🎬 A demo project for The Movie DB based on Kotlin MVVM architecture and material design & animations.
https://github.com/skydoves/themovies
🎬 A demo project for The Movie DB based on Kotlin MVVM architecture and material design & animations. - skydoves/TheMovies
- 543An alternative YouTube front-end
https://github.com/zt64/Hyperion
An alternative YouTube front-end. Contribute to zt64/Hyperion development by creating an account on GitHub.
- 544Android fragment stack controller
https://github.com/inshiro/Skate
Android fragment stack controller. Contribute to inshiro/skate development by creating an account on GitHub.
- 545Kotlin System Dynamics Toolkit
https://github.com/unipu-ict/ksdtoolkit
Kotlin System Dynamics Toolkit. Contribute to unipu-ict/ksdtoolkit development by creating an account on GitHub.
- 546Corda is an open source blockchain project, designed for business from the start. Only Corda allows you to build interoperable blockchain networks that transact in strict privacy. Corda's smart contract technology allows businesses to transact directly, with value.
https://github.com/corda/corda
Corda is an open source blockchain project, designed for business from the start. Only Corda allows you to build interoperable blockchain networks that transact in strict privacy. Corda's smart...
- 547Image loading for Android and Compose Multiplatform.
https://github.com/coil-kt/coil
Image loading for Android and Compose Multiplatform. - coil-kt/coil
- 548Kotlin Unit Testing Examples
https://github.com/rozkminiacz/KotlinUnitTesting
Kotlin Unit Testing Examples. Contribute to rozkminiacz/KotlinUnitTesting development by creating an account on GitHub.
- 549Mobile client for official Nextcloud News App written as Kotlin Multiplatform Project
https://github.com/SimonSchubert/NewsOut
Mobile client for official Nextcloud News App written as Kotlin Multiplatform Project - SimonSchubert/Newsout
- 550A Bluetooth kotlin multiplatform "Cross-Platform" library for iOS and Android
https://github.com/Reedyuk/blue-falcon
A Bluetooth kotlin multiplatform "Cross-Platform" library for iOS and Android - Reedyuk/blue-falcon
- 551Gradle Kotlin (http://kotlinlang.org) plugin for frontend development
https://github.com/Kotlin/kotlin-frontend-plugin
Gradle Kotlin (http://kotlinlang.org) plugin for frontend development - Kotlin/kotlin-frontend-plugin
- 552Android Library for requesting Permissions with Kotlin Flow
https://github.com/deva666/peko
Android Library for requesting Permissions with Kotlin Flow - deva666/Peko
- 553🎶 Chromatic tuner app for Android
https://github.com/adrielcafe/ChromaAndroidApp
🎶 Chromatic tuner app for Android. Contribute to adrielcafe/chroma development by creating an account on GitHub.
- 554[DEPRECATED] 🔥 Unofficial Kotlin Extensions for the Firebase Android SDK.
https://github.com/rosariopfernandes/fireXtensions
[DEPRECATED] 🔥 Unofficial Kotlin Extensions for the Firebase Android SDK. - thatfiredev/fireXtensions
- 555An object oriented toolkit for MongoDB Stitch in KotlinJS
https://github.com/fortytwoapps/kstitch
An object oriented toolkit for MongoDB Stitch in KotlinJS - robert-cronin/kstitch
- 556A fork of our clean architecture boilerplate using the Model-View-Intent pattern
https://github.com/bufferapp/android-clean-architecture-mvi-boilerplate
A fork of our clean architecture boilerplate using the Model-View-Intent pattern - bufferapp/android-clean-architecture-mvi-boilerplate
- 557💡🚀⭐️ A generalized adapter for RecyclerView on Android which makes it easy to add heterogeneous items to a list
https://github.com/rahulchowdhury/Mystique
💡🚀⭐️ A generalized adapter for RecyclerView on Android which makes it easy to add heterogeneous items to a list - rahulchowdhury/Mystique
- 558Bloat-free Immediate Mode Graphical User interface for JVM with minimal dependencies (rewrite of dear imgui)
https://github.com/kotlin-graphics/imgui
Bloat-free Immediate Mode Graphical User interface for JVM with minimal dependencies (rewrite of dear imgui) - kotlin-graphics/imgui
- 559Repository with source code from http://rosettacode.org/wiki/Category:Kotlin
https://github.com/dkandalov/rosettacode-kotlin
Repository with source code from http://rosettacode.org/wiki/Category:Kotlin - dkandalov/rosettacode-kotlin
- 560Advancement Utils for Android Developer written in Kotlin
https://github.com/WindSekirun/RichUtilsKt
Advancement Utils for Android Developer written in Kotlin - WindSekirun/RichUtilsKt
- 561Kotlin/Native interop to libui: a portable GUI library
https://github.com/msink/kotlin-libui
Kotlin/Native interop to libui: a portable GUI library - msink/kotlin-libui
- 562❤️ A sample Marvel heroes application based on MVVM (ViewModel, Coroutines, Room, Repository, Koin) architecture.
https://github.com/skydoves/MarvelHeroes
❤️ A sample Marvel heroes application based on MVVM (ViewModel, Coroutines, Room, Repository, Koin) architecture. - skydoves/MarvelHeroes
- 563An library to help android developers working easly with activities and fragments (Kotlin version)
https://github.com/massivedisaster/AFM
An library to help android developers working easly with activities and fragments (Kotlin version) - massivedisaster/AFM
- 564This is a work-in-progress (🔧️) ultraviolet index viewer app for demonstrating Instant Apps + Kotlin + Dagger + MVP
https://github.com/mustafaberkaymutlu/uv-index
This is a work-in-progress (🔧️) ultraviolet index viewer app for demonstrating Instant Apps + Kotlin + Dagger + MVP - mustafaberkaymutlu/uv-index
- 565Comparison among Java, Groovy, Scala, Kotlin in Android Development.
https://github.com/SidneyXu/AndroidDemoIn4Languages
Comparison among Java, Groovy, Scala, Kotlin in Android Development. - SidneyXu/AndroidDemoIn4Languages
- 566A small framework for writing client-side web-applications in Kotlin
https://github.com/stangls/kotlin-js-jquery
A small framework for writing client-side web-applications in Kotlin - stangls/kotlin-js-jquery
- 567lightningkite/kotlin-core
https://github.com/lightningkite/kotlin-core
Contribute to lightningkite/kotlin-core development by creating an account on GitHub.
- 568Kotlin native with SFML example
https://github.com/perses-games/konan-sfml
Kotlin native with SFML example. Contribute to perses-games/konan-sfml development by creating an account on GitHub.
- 569Kotlin TodoMVC – full-stack Kotlin application demo
https://github.com/gyulavoros/kotlin-todomvc
Kotlin TodoMVC – full-stack Kotlin application demo - gyulavoros/kotlin-todomvc
- 570Scans and solves Sudoku Puzzles from images using AI
https://github.com/pintowar/sudoscan
Scans and solves Sudoku Puzzles from images using AI - pintowar/sudoscan
- 571🍞 The ultimate breadcrumbs view for Android!
https://github.com/adrielcafe/KrumbsView
🍞 The ultimate breadcrumbs view for Android! Contribute to adrielcafe/krumbsview development by creating an account on GitHub.
- 572A growing library of assorted data structures, algorithms and utilities for OPENRNDR
https://github.com/openrndr/orx
A growing library of assorted data structures, algorithms and utilities for OPENRNDR - openrndr/orx
- 573jvm-graphics-labs/learn-OpenGL
https://github.com/java-opengl-labs/learn-OpenGL
Contribute to jvm-graphics-labs/learn-OpenGL development by creating an account on GitHub.
- 574Easy Android permissions. Powered by Kotlin.
https://github.com/mr-wizman/Consent
Easy Android permissions. Powered by Kotlin. Contribute to simplisticated/Consent development by creating an account on GitHub.
- 575An easy and flexible way to implement loading skeletons and shimmering effects on layouts for Android.
https://github.com/skydoves/AndroidVeil
:performing_arts: An easy and flexible way to implement loading skeletons and shimmering effects on layouts for Android. - GitHub - skydoves/AndroidVeil: An easy and flexible way to implement load...
- 576Unofficial companion app for the game Green Hell
https://github.com/adrielcafe/GreenHellCompanionApp
Unofficial companion app for the game Green Hell. Contribute to adrielcafe/GreenHellCompanionApp development by creating an account on GitHub.
- 577Android Kotlin Examples
https://github.com/inaka/kotlillon
Android Kotlin Examples. Contribute to inaka/kotlillon development by creating an account on GitHub.
- 578KataContacts written in Kotlin. The main goal is to practice Clean Architecture Development
https://github.com/Karumi/KataContactsKotlin
KataContacts written in Kotlin. The main goal is to practice Clean Architecture Development - Karumi/KataContactsKotlin
- 579Anxiety free news reader for Android - developed using Kotlin
https://github.com/dodyg/AndroidRivers
Anxiety free news reader for Android - developed using Kotlin - dodyg/AndroidRivers
- 580Powerfull Collections, Sets, Lists and Maps.
https://github.com/Poweranimal/PowerCollections
Powerfull Collections, Sets, Lists and Maps. Contribute to Poweranimal/PowerCollections development by creating an account on GitHub.
- 581A Kotlin-based build system for the JVM.
https://github.com/cbeust/kobalt
A Kotlin-based build system for the JVM. Contribute to cbeust/kobalt development by creating an account on GitHub.
- 582Kotpref - Android SharedPreferences delegation library for Kotlin
https://github.com/chibatching/Kotpref
Kotpref - Android SharedPreferences delegation library for Kotlin - chibatching/Kotpref
- 583StaticLog - super lightweight static logging for Kotlin, Java and Android
https://github.com/jupf/staticlog
StaticLog - super lightweight static logging for Kotlin, Java and Android - jupf/staticlog
- 584🎬 Modern powerful TMDB API to fetch movies and TV shows for Kotlin Multiplatform.
https://github.com/MoviebaseApp/tmdb-api
🎬 Modern powerful TMDB API to fetch movies and TV shows for Kotlin Multiplatform. - GitHub - MoviebaseApp/tmdb-kotlin: 🎬 Modern powerful TMDB API to fetch movies and TV shows for Kotlin Multiplatf...
- 585A Kotlin work manager library for Android with progress notifications and Hilt support.
https://github.com/evilthreads669966/BootLaces
A Kotlin work manager library for Android with progress notifications and Hilt support. - evilthreads669966/BootLaces
- 586Kotlin wrappers for Three.js
https://github.com/markaren/three-kt-wrapper
Kotlin wrappers for Three.js . Contribute to markaren/three-kt-wrapper development by creating an account on GitHub.
- 587Android application compatible with ZX2C4's Pass command line application
https://github.com/android-password-store/Android-Password-Store
Android application compatible with ZX2C4's Pass command line application - android-password-store/Android-Password-Store
- 588Easily create views with a background of repeating dashes 🐝
https://github.com/MackHartley/DashedView
Easily create views with a background of repeating dashes 🐝 - MackHartley/DashedView
- 589🔎 An HTTP inspector for Android & OkHTTP (like Charles but on device)
https://github.com/ChuckerTeam/chucker
🔎 An HTTP inspector for Android & OkHTTP (like Charles but on device) - ChuckerTeam/chucker
- 590A simple library to add custom toast to android applications.
https://github.com/developingdeveloper-tech/toaster-android
A simple library to add custom toast to android applications. - 5AbhishekSaxena/toaster-android
- 591Kotlin bindings for JS libraries.
https://github.com/danfma/kodando
Kotlin bindings for JS libraries. Contribute to kodando/kodando development by creating an account on GitHub.
- 592欢乐21点
https://github.com/ahong222/happy21
欢乐21点. Contribute to ahong222/happy21 development by creating an account on GitHub.
- 593ts2kt is officially deprecated, please use https://github.com/Kotlin/dukat instead. // Converter of TypeScript definition files to Kotlin external declarations
https://github.com/kotlin/ts2kt
ts2kt is officially deprecated, please use https://github.com/Kotlin/dukat instead. // Converter of TypeScript definition files to Kotlin external declarations - Kotlin/ts2kt
- 594Let's Learn Kotlin Programming 💜 🧡
https://github.com/halilozel1903/LearnKotlinProgramming
Let's Learn Kotlin Programming 💜 🧡. Contribute to halilozel1903/LearnKotlinProgramming development by creating an account on GitHub.
- 595A simple way to handle remote image in Kotlin.
https://github.com/matteocrippa/Parrot
A simple way to handle remote image in Kotlin. Contribute to matteocrippa/Parrot development by creating an account on GitHub.
- 596Free and open source Bible for Android
https://github.com/inshiro/Kodesh
Free and open source Bible for Android. Contribute to inshiro/kodesh development by creating an account on GitHub.
- 597Gradle plugin to help with build and test of Jenkins Pipeline Shared Libraries (see https://jenkins.io/doc/book/pipeline/shared-libraries/)
https://github.com/mkobit/jenkins-pipeline-shared-libraries-gradle-plugin
Gradle plugin to help with build and test of Jenkins Pipeline Shared Libraries (see https://jenkins.io/doc/book/pipeline/shared-libraries/) - mkobit/jenkins-pipeline-shared-libraries-gradle-plugin
- 598🏺Create a PKCS12 TrustStore by retrieving server certificates.
https://github.com/sureshg/InstallCerts
🏺Create a PKCS12 TrustStore by retrieving server certificates. - sureshg/InstallCerts
- 599Auto-generate Parcelable implementations for Java and Kotlin
https://github.com/grandstaish/paperparcel
Auto-generate Parcelable implementations for Java and Kotlin - grandstaish/paperparcel
- 600A simple 'Slide to Unlock' Material widget for Android, written in Kotlin 📱🎨🦄
https://github.com/cortinico/slidetoact
A simple 'Slide to Unlock' Material widget for Android, written in Kotlin 📱🎨🦄 - cortinico/slidetoact
- 601Simple Android Library, that provides easy way to start the Activities with arguments.
https://github.com/MarcinMoskala/ActivityStarter
Simple Android Library, that provides easy way to start the Activities with arguments. - MarcinMoskala/ActivityStarter
- 602Simple server for proxy requests and host static files written in Kotlin and Undertow.
https://github.com/IRus/kotlin-dev-proxy
Simple server for proxy requests and host static files written in Kotlin and Undertow. - Heapy/kotlin-dev-proxy
- 603🚀 Plugin for Android Studio And IntelliJ Idea to generate Kotlin data class code from JSON text ( Json to Kotlin )
https://github.com/wuseal/JsonToKotlinClass
🚀 Plugin for Android Studio And IntelliJ Idea to generate Kotlin data class code from JSON text ( Json to Kotlin ) - wuseal/JsonToKotlinClass
- 604Easy Android logging with Kotlin and Timber
https://github.com/ajalt/timberkt
Easy Android logging with Kotlin and Timber. Contribute to ajalt/timberkt development by creating an account on GitHub.
- 605Lmgtfy Generator Open Source Android App - built to learn Kotlin, the MVP architecture, Dagger and Rx
https://github.com/WilderPereira/lmgtfyGen
Lmgtfy Generator Open Source Android App - built to learn Kotlin, the MVP architecture, Dagger and Rx - WilderPereira/lmgtfyGen
- 606Minimal framework for Model View Intent inspired Android applications written in Kotlin
https://github.com/hpost/rx-mvi
Minimal framework for Model View Intent inspired Android applications written in Kotlin - hpost/rx-mvi
- 607A native version of pixi
https://github.com/pixijs/pixi-native
A native version of pixi. Contribute to pixijs/pixi-native development by creating an account on GitHub.
- 608Develop browser games in Kotlin
https://github.com/perses-games/kudens
Develop browser games in Kotlin. Contribute to perses-games/kudens development by creating an account on GitHub.
- 609OkHttp Kotlin command line
https://github.com/yschimke/okurl
OkHttp Kotlin command line. Contribute to yschimke/okurl development by creating an account on GitHub.
- 610It's a project that contains lessons & examples about Kotlin Programming language. 🏜️
https://github.com/halilozel1903/KotlinTutorials
It's a project that contains lessons & examples about Kotlin Programming language. 🏜️ - halilozel1903/KotlinTutorials
- 611An Android, JavaFx, JS multiplatform datavisualization library with comprehensive DSL
https://github.com/data2viz/data2viz
An Android, JavaFx, JS multiplatform datavisualization library with comprehensive DSL - data2viz/data2viz
- 612Plugin for publishing JVM software through NPM.
https://github.com/neworld/gradle-jdeploy-plugin
Plugin for publishing JVM software through NPM. . Contribute to neworld/gradle-jdeploy-plugin development by creating an account on GitHub.
- 613K5-compose is a sketchy port of p5.js for Jetpack Compose Desktop
https://github.com/CuriousNikhil/k5-compose
K5-compose is a sketchy port of p5.js for Jetpack Compose Desktop - CuriousNikhil/k5-compose
- 614🍭 Candy Crush Clone
https://github.com/TobseF/Candy-Crush-Clone
🍭 Candy Crush Clone. Contribute to TobseF/Candy-Crush-Clone development by creating an account on GitHub.
- 615Build software better, together
https://github.com/MohsinAli0899/Book
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 6161M+ downloads Linux reference app with basics, tips and formatted man pages
https://github.com/SimonSchubert/LinuxCommandBibliotheca
1M+ downloads Linux reference app with basics, tips and formatted man pages - SimonSchubert/LinuxCommandLibrary
- 617A Kotlin Android library for forensics evasion & heuristics evasion that prevents your code from being tested.
https://github.com/evilthreads669966/EvadeMe
A Kotlin Android library for forensics evasion & heuristics evasion that prevents your code from being tested. - evilthreads669966/EvadeMe
- 618A collection of useful Kotlin extension for Android
https://github.com/matteocrippa/karamba
A collection of useful Kotlin extension for Android - matteocrippa/karamba
- 619TODO API Client Kata for Kotlin Developers. The main goal is to practice integration testing using MockWebServer
https://github.com/Karumi/KataTODOApiClientKotlin
TODO API Client Kata for Kotlin Developers. The main goal is to practice integration testing using MockWebServer - Karumi/KataTODOApiClientKotlin
- 620Configurable BDD testing using a Kotlin DSL for Gherkin
https://github.com/som-one/zarif-kherkin
Configurable BDD testing using a Kotlin DSL for Gherkin - som-one/zarif-kherkin
- 621Nice and simple DSL for Espresso Compose UI testing in Kotlin
https://github.com/KakaoCup/Compose
Nice and simple DSL for Espresso Compose UI testing in Kotlin - KakaoCup/Compose
- 622An extremely barebones boilerplate project for compiling Kotlin to Javascript
https://github.com/kengorab/kotlin-javascript-boilerplate
An extremely barebones boilerplate project for compiling Kotlin to Javascript - kengorab/kotlin-javascript-boilerplate
- 623🦁 A Disney app using transformation motions based on MVVM (ViewModel, Coroutines, Flow, Room, Repository, Koin) architecture.
https://github.com/skydoves/DisneyMotions
🦁 A Disney app using transformation motions based on MVVM (ViewModel, Coroutines, Flow, Room, Repository, Koin) architecture. - skydoves/DisneyMotions
- 624:camera: Easy Photo Map is a photomap application that displays the location of the photo on the map using the location information included in the photo.
https://github.com/hanjoongcho/aaf-easyphotomap
:camera: Easy Photo Map is a photomap application that displays the location of the photo on the map using the location information included in the photo. - hanjoongcho/aaf-easyphotomap
- 625Android app to help to tune a banjo
https://github.com/MakinGiants/banjen
Android app to help to tune a banjo. Contribute to MakinGiants/banjen development by creating an account on GitHub.
- 626Android TextToSpeech Helper to talk any text you want and handle events.
https://github.com/CuriousNikhil/gossip
Android TextToSpeech Helper to talk any text you want and handle events. - CuriousNikhil/gossip
- 627Kotlin logging, both js and jvm
https://github.com/shafirov/klogging
Kotlin logging, both js and jvm. Contribute to shafirov/klogging development by creating an account on GitHub.
- 628Kotlin/JS bindings for PIXI.js library.
https://github.com/Ayfri/PIXI-Kotlin
Kotlin/JS bindings for PIXI.js library. Contribute to Ayfri/PIXI-Kotlin development by creating an account on GitHub.
- 629:lock: Easy Password is password management application. This application uses pattern locks to manage information that requires security.
https://github.com/hanjoongcho/aaf-easypassword
:lock: Easy Password is password management application. This application uses pattern locks to manage information that requires security. - hanjoongcho/aaf-easypassword
- 630This is a first kotlin project
https://github.com/RxKotlin/Pocket
This is a first kotlin project. Contribute to RxKotlin/Pocket development by creating an account on GitHub.
- 631RHRE – A playable, custom remix editor for the Rhythm Heaven series
https://github.com/chrislo27/RhythmHeavenRemixEditor
RHRE – A playable, custom remix editor for the Rhythm Heaven series - chrislo27/RhythmHeavenRemixEditor
- 632Easy to use and concise yet powerful and robust command line argument parsing for Kotlin
https://github.com/xenomachina/kotlin-argparser
Easy to use and concise yet powerful and robust command line argument parsing for Kotlin - xenomachina/kotlin-argparser
- 633Build software better, together
https://github.com/evilthreads669966/EasyShells
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 634Create kotlin android project with one line of command.
https://github.com/nekocode/kotgo
Create kotlin android project with one line of command. - nekocode/create-android-kotlin-app
- 635🎓 Learning Kotlin Coroutines and Flows for Android by example. 🚀 Sample implementations for real-world Android use cases. 🛠 Unit tests included!
https://github.com/LukasLechnerDev/Kotlin-Coroutine-Use-Cases-on-Android
🎓 Learning Kotlin Coroutines and Flows for Android by example. 🚀 Sample implementations for real-world Android use cases. 🛠 Unit tests included! - LukasLechnerDev/Kotlin-Coroutines-and-Flow-UseCase...
- 636The Lazybones app migrated to Kotlin as a learning exercise
https://github.com/robfletcher/lazybones-kotlin
The Lazybones app migrated to Kotlin as a learning exercise - robfletcher/lazybones-kotlin
- 637Maxibon kata for Kotlin Developers. The main goal is to practice property based testing.
https://github.com/Karumi/MaxibonKataKotlin
Maxibon kata for Kotlin Developers. The main goal is to practice property based testing. - Karumi/MaxibonKataKotlin
- 638❤️ A heart-shaped toggle switch component for Jetpack Compose
https://github.com/popovanton0/heart-switch
❤️ A heart-shaped toggle switch component for Jetpack Compose - popovanton0/heart-switch
- 639Converter of <any kind of declarations> to Kotlin external declarations
https://github.com/Kotlin/dukat
Converter of <any kind of declarations> to Kotlin external declarations - Kotlin/dukat
- 640A Kotlin Android library for content provider queries with reactive streams and coroutines.
https://github.com/evilthreads669966/Pickpocket
A Kotlin Android library for content provider queries with reactive streams and coroutines. - evilthreads669966/Pickpocket
- 641A multi-paradigm programming language running on JVM
https://github.com/lice-lang/lice
A multi-paradigm programming language running on JVM - lice-lang/lice
- 642🔴 A non-deterministic finite-state machine for Android & JVM that won't let you down
https://github.com/adrielcafe/HAL
🔴 A non-deterministic finite-state machine for Android & JVM that won't let you down - adrielcafe/hal
- 643A customizable, animated progress bar that features rounded corners. This Android library is designed to look great and be simple to use 🎉
https://github.com/MackHartley/RoundedProgressBar
A customizable, animated progress bar that features rounded corners. This Android library is designed to look great and be simple to use 🎉 - MackHartley/RoundedProgressBar
- 644Libraries and tools supporting the use of Vue.js in Kotlin. (This project is finished. There are no plans to update in the future. )
https://github.com/nosix/vue-kotlin
Libraries and tools supporting the use of Vue.js in Kotlin. (This project is finished. There are no plans to update in the future. ) - nosix/vue-kotlin
- 645Jenkins plugin for automated documentation uploading to Confluence.
https://github.com/jenkinsci/doktor-plugin
Jenkins plugin for automated documentation uploading to Confluence. - jenkinsci/doktor-plugin
- 646Kotlin DSL for CSS
https://github.com/olegcherr/Aza-Kotlin-CSS
Kotlin DSL for CSS. Contribute to olegcherr/Aza-Kotlin-CSS development by creating an account on GitHub.
- 647Showcase project of Functional Reactive Programming on Android, using RxJava.
https://github.com/pakoito/FunctionalAndroidReference
Showcase project of Functional Reactive Programming on Android, using RxJava. - pakoito/FunctionalAndroidReference
- 648A simple, coroutine based Kotlin Email API for both client- and server-side projects
https://github.com/bluefireoly/SimpleKotlinMail
A simple, coroutine based Kotlin Email API for both client- and server-side projects - jakobkmar/SimpleKotlinMail
- 649🚀Optimizer for mobile applications
https://github.com/didi/booster
🚀Optimizer for mobile applications. Contribute to didi/booster development by creating an account on GitHub.
- 650Java/Kotlin compiled code viewer
https://github.com/borisf/classyshark-bytecode-viewer
Java/Kotlin compiled code viewer. Contribute to borisf/classyshark-bytecode-viewer development by creating an account on GitHub.
- 651Object oriented web framework for Kotlin/JS
https://github.com/rjaros/kvision
Object oriented web framework for Kotlin/JS. Contribute to rjaros/kvision development by creating an account on GitHub.
- 652Easy to use cryptographic framework for data protection: secure messaging with forward secrecy and secure data storage. Has unified APIs across 14 platforms.
https://github.com/cossacklabs/themis
Easy to use cryptographic framework for data protection: secure messaging with forward secrecy and secure data storage. Has unified APIs across 14 platforms. - cossacklabs/themis
- 653🍲Foodium is a sample food blog Android application 📱 built to demonstrate the use of Modern Android development tools - (Kotlin, Coroutines, Flow, Dagger 2/Hilt, Architecture Components, MVVM, Room, Retrofit, Moshi, Material Components).
https://github.com/PatilShreyas/Foodium
🍲Foodium is a sample food blog Android application 📱 built to demonstrate the use of Modern Android development tools - (Kotlin, Coroutines, Flow, Dagger 2/Hilt, Architecture Components, MVVM, Roo...
- 654🦄 Sourcerer app makes a visual profile from your GitHub and git repositories.
https://github.com/sourcerer-io/sourcerer-app
🦄 Sourcerer app makes a visual profile from your GitHub and git repositories. - sourcerer-io/sourcerer-app
- 655🌈Rainbow Brackets for IntelliJ based IDEs/Android Studio/HUAWEI DevEco Studio/Fleet
https://github.com/izhangzhihao/intellij-rainbow-brackets
🌈Rainbow Brackets for IntelliJ based IDEs/Android Studio/HUAWEI DevEco Studio/Fleet - izhangzhihao/intellij-rainbow-brackets
- 656Beautiful and feature rich workout log app.
https://github.com/AnkitSuda/Rebound
Beautiful and feature rich workout log app. Contribute to AnkitSuda/Rebound development by creating an account on GitHub.
- 657:blowfish: An Android & JVM key-value storage powered by Protobuf and Coroutines
https://github.com/adrielcafe/PufferDB
:blowfish: An Android & JVM key-value storage powered by Protobuf and Coroutines - adrielcafe/pufferdb
- 658Music Player GO - Apps on Google Play
https://play.google.com/store/apps/details?id=com.iven.musicplayergo
Very simple, nice, privacy-friendly and original local Android Music Player!
- 659Examples and demos for the new Vulkan API
https://github.com/java-opengl-labs/Vulkan
Examples and demos for the new Vulkan API . Contribute to jvm-graphics-labs/Vulkan development by creating an account on GitHub.
- 660🕸️ Graphs, finite fields and discrete dynamical systems in Kotlin
https://github.com/breandan/kaliningraph
🕸️ Graphs, finite fields and discrete dynamical systems in Kotlin - breandan/galoisenne
- 661Bazel rules for Kotlin
https://github.com/pubref/rules_kotlin
Bazel rules for Kotlin. Contribute to pubref/rules_kotlin development by creating an account on GitHub.
- 662:blue_book: A diary application optimized for user experience.
https://github.com/hanjoongcho/aaf-easydiary
:blue_book: A diary application optimized for user experience. - hanjoongcho/aaf-easydiary
- 663🍼Debug Bottle is an Android runtime debug / develop tools written using kotlin language.
https://github.com/kiruto/debug-bottle
🍼Debug Bottle is an Android runtime debug / develop tools written using kotlin language. - kiruto/debug-bottle
- 664A boilerplate-free Kotlin config library for loading configuration files as data classes
https://github.com/sksamuel/hoplite
A boilerplate-free Kotlin config library for loading configuration files as data classes - sksamuel/hoplite
- 665GameBoy Emulator Environment / Front-end - Kotlin
https://github.com/vicboma1/GameBoyEmulatorEnvironment
GameBoy Emulator Environment / Front-end - Kotlin - GitHub - vicboma1/GameBoyEmulatorEnvironment: GameBoy Emulator Environment / Front-end - Kotlin
- 666Various examples for Kotlin
https://github.com/JetBrains/kotlin-examples
Various examples for Kotlin. Contribute to Kotlin/kotlin-examples development by creating an account on GitHub.
- 667📚 Sample Android Components Architecture on a modular word focused on the scalability, testability and maintainability written in Kotlin, following best practices using Jetpack.
https://github.com/VMadalin/kotlin-sample-app
📚 Sample Android Components Architecture on a modular word focused on the scalability, testability and maintainability written in Kotlin, following best practices using Jetpack. - vmadalin/android...
- 668The Android maps adapter
https://github.com/TradeMe/MapMe
The Android maps adapter. Contribute to TradeMe/MapMe development by creating an account on GitHub.
- 669A lightweight Android activity router
https://github.com/denisidoro/krouter
A lightweight Android activity router. Contribute to denisidoro/krouter development by creating an account on GitHub.
- 670(Deprecated) Kotlin Native/JS/JVM Annotation Processor library for Kotlin compiler plugins
https://github.com/Foso/MpApt
(Deprecated) :wrench: Kotlin Native/JS/JVM Annotation Processor library for Kotlin compiler plugins - GitHub - Foso/MpApt: (Deprecated) Kotlin Native/JS/JVM Annotation Processor library for Kotlin ...
- 671Make a cool intro for your Android app.
https://github.com/AppIntro/AppIntro
Make a cool intro for your Android app. Contribute to AppIntro/AppIntro development by creating an account on GitHub.
- 672It's finally easy to take photos/videos via camera or get photos/videos from gallery on Android.
https://github.com/levibostian/Shutter-Android
It's finally easy to take photos/videos via camera or get photos/videos from gallery on Android. - GitHub - levibostian/Shutter-Android: It's finally easy to take photos/videos via camera ...
- 673Minimal UI library for Android inspired by React
https://github.com/zserge/anvil
Minimal UI library for Android inspired by React. Contribute to anvil-ui/anvil development by creating an account on GitHub.
- 674Kotlin/kotlinconf-spinner
https://github.com/JetBrains/kotlinconf-spinner
Contribute to Kotlin/kotlinconf-spinner development by creating an account on GitHub.
Related Articlesto learn about angular.
- 1Kotlin 101: Beginner’s Guide to Modern Programming
- 2Kotlin Data Types and Variables: Beginner’s Guide
- 3Kotlin’s Powerful Null Safety Features
- 4Higher-Order Functions and Lambdas in Kotlin
- 5Android Apps with Kotlin: Step-by-Step Guide
- 6Jetpack Compose: Building Modern Android UIs with Kotlin
- 7Building RESTful APIs with Kotlin and Ktor: Practical Guide
- 8Kotlin for Microservices: Building Scalable Backend Systems
- 9Kotlin Coroutines: Simplifying Asynchronous Programming
- 10Kotlin Multiplatform: Building Cross-Platform Apps with Shared Code
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here 🔍.
- 1
how to use kotlin programming language
- 2
who created kotlin
- 3
should i learn kotlin or java
- 4
how to use kotlin
- 5
when was kotlin programming language created
- 6
is kotlin better than python
- 7
what is kotlin programming language
- 8
can kotlin use java libraries
- 9
when was kotlin released
- 10
does kotlin require java
- 11
why kotlin is bad
- 12
how to pronounce kotlin
- 13
does kotlin run on jvm
- 14
- 15
what is kotlin and why use it
- 16
who invented kotlin programming language
- 17
is kotlin hard to learn
- 18
is kotlin written in java
- 19
- 20
does kotlin compile to java
- 21
- 22
when did kotlin come out
- 23
who uses kotlin
- 24
what is kotlin good for
- 25
when kotlin example
- 26
what is kotlin programming
- 27
when kotlin default
- 28
do while kotlin
- 29
what is kotlin programming language used for
- 30
who invented kotlin
- 31
is kotlin good for competitive programming
- 32
is kotlin functional programming
- 33
is kotlin the future
- 34
what is functional programming in kotlin
- 35
should i learn kotlin
- 36
- 37
is kotlin based on java
- 38
can java call kotlin
- 39
how to learn kotlin programming language
- 40
who made kotlin
- 41
how to program in kotlin
- 42
who owns kotlin
- 43
why was kotlin created
- 44
is kotlin worth learning
- 45
where kotlin is used
- 46
can kotlin replace java
- 47
will kotlin replace java reddit
- 48
why kotlin vs java
- 49
does kotlin use java
- 50
is kotlin programming language
- 51
is kotlin functional programming language
- 52
does kotlin replace java
- 53
who created kotlin language
- 54
is kotlin statically typed programming language
- 55
should i learn kotlin or java for android development
- 56
is kotlin good for beginners
- 57
will kotlin replace java
- 58
where kotlin is implemented
- 59
why learn kotlin
- 60
what can kotlin be used for
- 61
can kotlin be used for backend
- 62
is kotlin popular
- 63
what is kotlin programming
- 64
- 65
why kotlin is used
- 66
when is kotlin created
- 67
how does kotlin work
More Sitesto check out once you're finished browsing here.
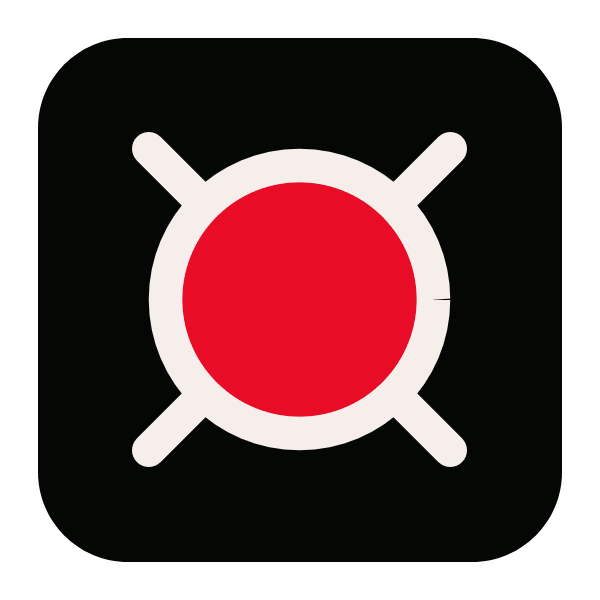
https://www.0x3d.site/
0x3d is designed for aggregating information.
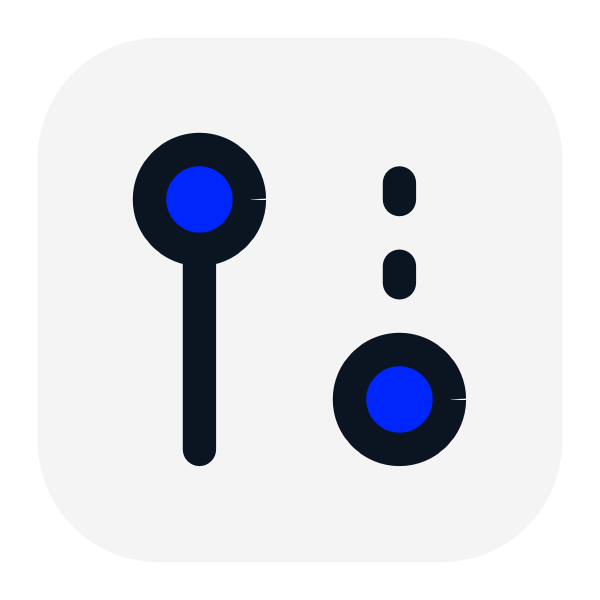
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
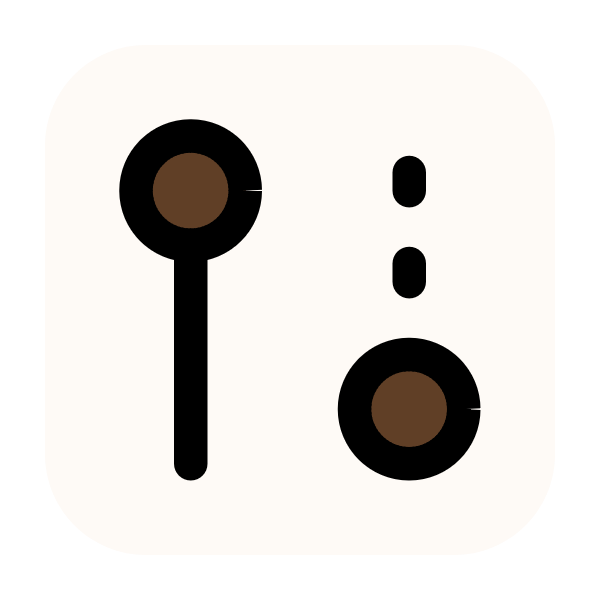
https://open-source.0x3d.site/
Open Source Online Directory
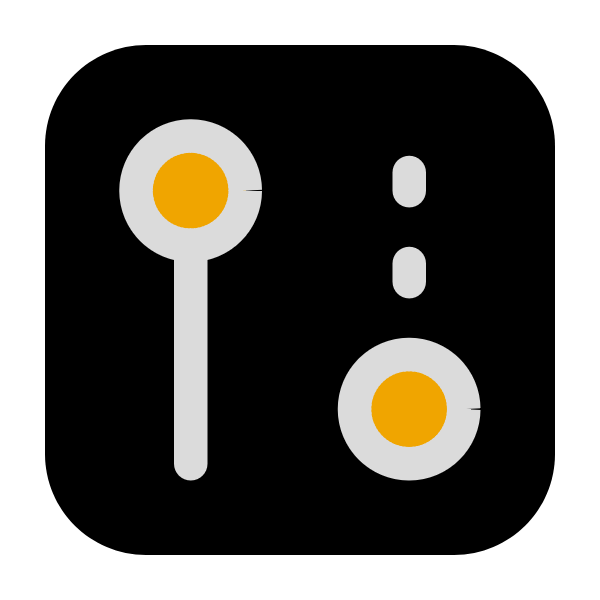
https://analytics.0x3d.site/
Analytics Online Directory
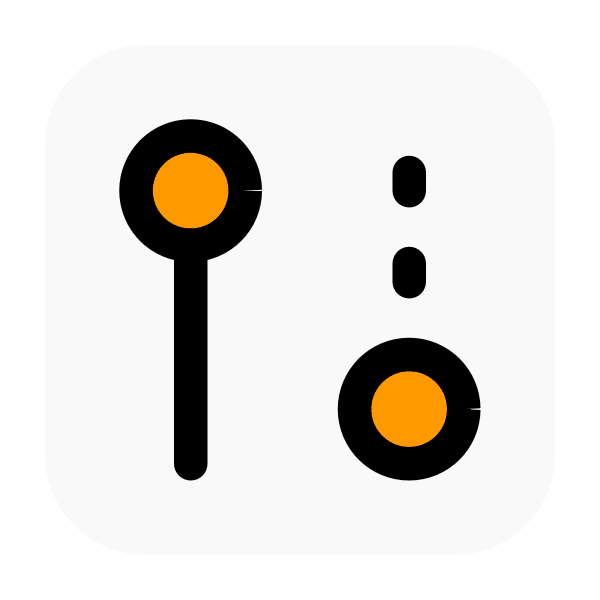
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
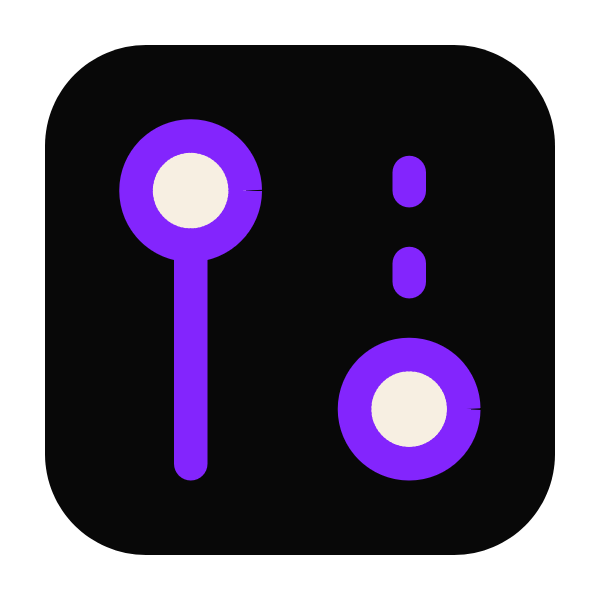
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
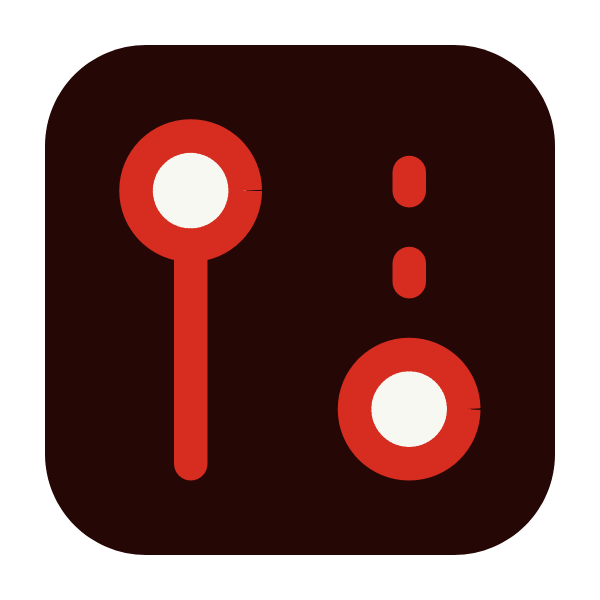
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
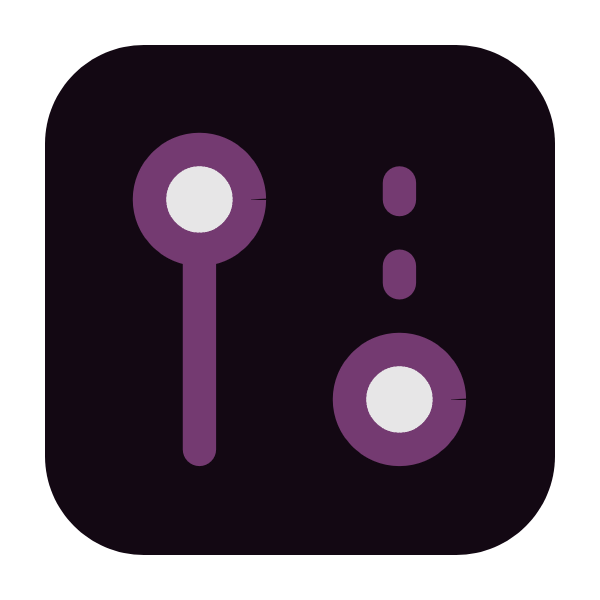
https://elixir.0x3d.site/
Elixir Online Directory
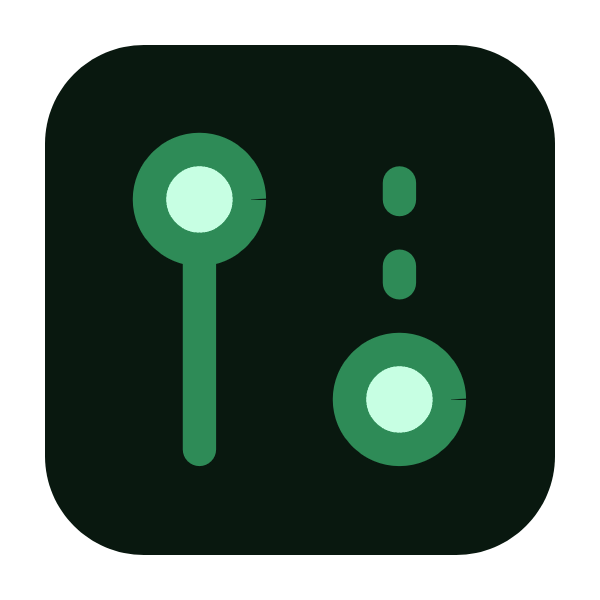
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
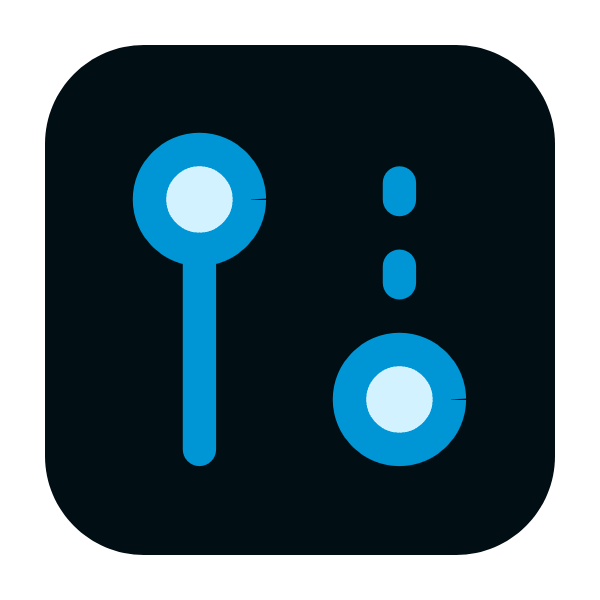
https://c-programming.0x3d.site/
C Programming Online Directory
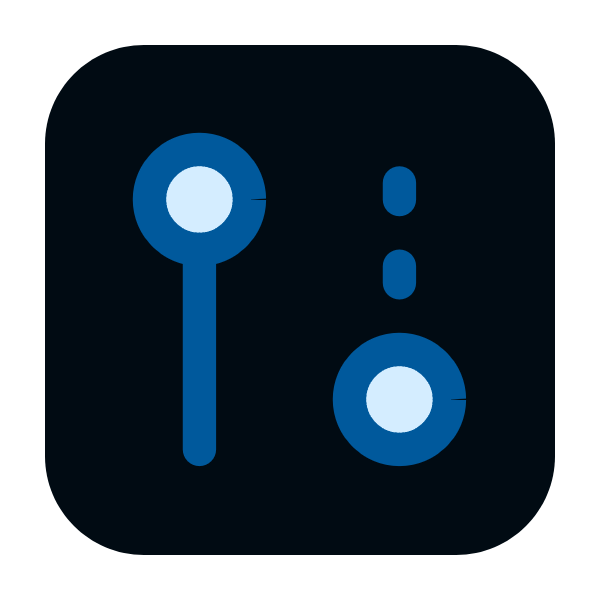
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
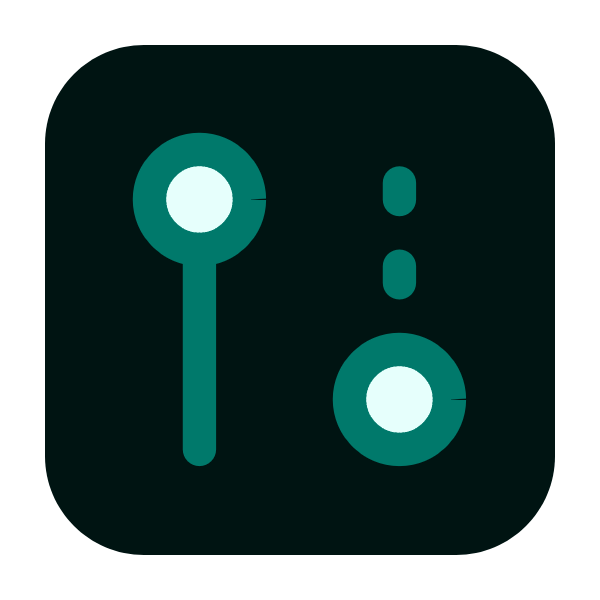
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
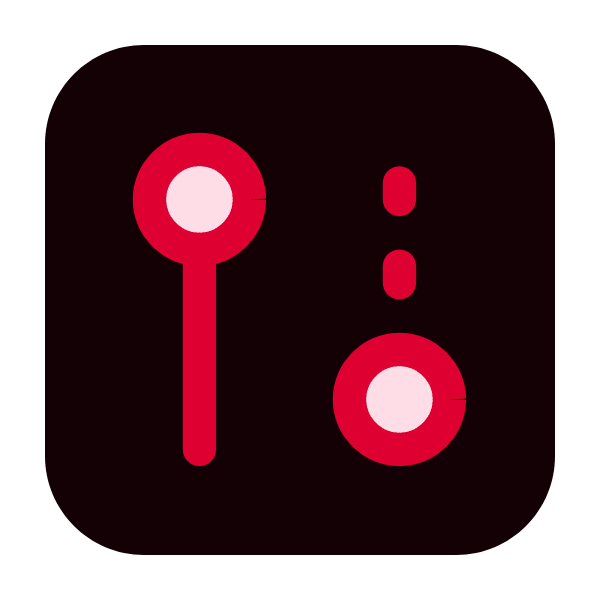
https://angular.0x3d.site/
Angular JS Online Directory